c++ 如何使用信号量挂起线程
时间: 2023-08-05 18:19:37 浏览: 115
在 C++ 中,可以使用 `std::mutex` 和 `std::condition_variable` 实现信号量来挂起线程。下面是一个简单的示例:
```c++
#include <mutex>
#include <condition_variable>
class Semaphore {
public:
Semaphore(int count = 0) : count_(count) {}
void notify() {
std::unique_lock<std::mutex> lock(mutex_);
++count_;
cv_.notify_one();
}
void wait() {
std::unique_lock<std::mutex> lock(mutex_);
while (count_ == 0) {
cv_.wait(lock);
}
--count_;
}
private:
std::mutex mutex_;
std::condition_variable cv_;
int count_;
};
```
在上面的示例中,`Semaphore` 类中包含了一个计数器 `count_`,并且提供了 `notify()` 和 `wait()` 两个方法,分别用于增加计数器和减少计数器并等待计数器不为零。当计数器为零时,线程会被挂起等待 `notify()` 方法的通知。
使用示例:
```c++
#include <iostream>
#include <thread>
Semaphore sem(0);
void worker() {
std::cout << "worker start" << std::endl;
sem.wait();
std::cout << "worker end" << std::endl;
}
int main() {
std::thread t(worker);
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "main notify" << std::endl;
sem.notify();
t.join();
return 0;
}
```
在上面的示例中,主线程会等待 1 秒后调用 `notify()` 方法通知子线程继续执行。子线程在 `wait()` 方法处被挂起,直到收到通知后才继续执行。输出结果为:
```
worker start
main notify
worker end
```
阅读全文
相关推荐





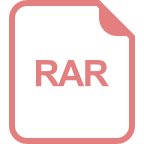
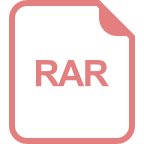





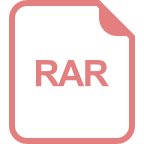





