py自定义列表四则运算并输出
时间: 2023-07-11 16:08:00 浏览: 73
可以通过自定义类来实现自定义列表的四则运算并输出。下面是一个简单的示例代码:
```python
class MyList:
def __init__(self, l):
self.lst = l
def __str__(self):
return str(self.lst)
def __add__(self, other):
if len(self.lst) != len(other.lst):
raise ValueError("Lists must have the same length")
result = [self.lst[i] + other.lst[i] for i in range(len(self.lst))]
return MyList(result)
def __sub__(self, other):
if len(self.lst) != len(other.lst):
raise ValueError("Lists must have the same length")
result = [self.lst[i] - other.lst[i] for i in range(len(self.lst))]
return MyList(result)
def __mul__(self, other):
if len(self.lst) != len(other.lst):
raise ValueError("Lists must have the same length")
result = [self.lst[i] * other.lst[i] for i in range(len(self.lst))]
return MyList(result)
def __truediv__(self, other):
if len(self.lst) != len(other.lst):
raise ValueError("Lists must have the same length")
result = [self.lst[i] / other.lst[i] for i in range(len(self.lst))]
return MyList(result)
# 测试
a = MyList([1, 2, 3])
b = MyList([4, 5, 6])
print(a + b) # 输出 [5, 7, 9]
print(a - b) # 输出 [-3, -3, -3]
print(a * b) # 输出 [4, 10, 18]
print(a / b) # 输出 [0.25, 0.4, 0.5]
```
在这个示例中,我们定义了一个名为 `MyList` 的类,它包含一个由列表 `lst` 组成的实例变量。我们还定义了 `__str__` 方法,以便在打印 `MyList` 对象时输出列表。然后,我们定义了四个运算符重载函数:`__add__`、`__sub__`、`__mul__` 和 `__truediv__`。这些函数实现了列表的加、减、乘和除运算,并返回一个新的 `MyList` 对象作为结果。
最后,我们在测试部分创建两个 `MyList` 对象 `a` 和 `b`,并对它们进行加、减、乘和除运算。结果被打印到控制台上。
相关推荐
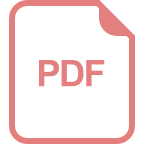














