用Python写一个银行管理系统
时间: 2024-03-30 17:17:03 浏览: 22
好的,以下是一个简单的银行管理系统的 Python 代码,包含基本的账户管理、存取款和查询功能:
```python
class BankAccount:
def __init__(self, name, balance=0.0):
self.name = name
self.balance = balance
def deposit(self, amount):
self.balance += amount
print("Deposited:", amount)
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
print("Withdrawn:", amount)
else:
print("Insufficient balance!")
def display(self):
print("Name:", self.name, "Balance:", self.balance)
accounts = {}
while True:
print("Bank Account Management System")
print("1. Create an account")
print("2. Deposit")
print("3. Withdraw")
print("4. Display account details")
print("5. Quit")
choice = int(input("Enter your choice: "))
if choice == 1:
name = input("Enter your name: ")
balance = float(input("Enter initial balance: "))
accounts[name] = BankAccount(name, balance)
print("Account created successfully!")
elif choice == 2:
name = input("Enter account holder name: ")
if name in accounts:
amount = float(input("Enter deposit amount: "))
accounts[name].deposit(amount)
else:
print("Account not found!")
elif choice == 3:
name = input("Enter account holder name: ")
if name in accounts:
amount = float(input("Enter withdrawal amount: "))
accounts[name].withdraw(amount)
else:
print("Account not found!")
elif choice == 4:
name = input("Enter account holder name: ")
if name in accounts:
accounts[name].display()
else:
print("Account not found!")
elif choice == 5:
break
else:
print("Invalid choice!")
```
当运行此程序时,它将显示一个菜单,用户可以选择创建账户、存款、取款、查询账户信息或退出程序。程序将使用字典来保存所有账户,并通过 BankAccount 类来管理每个账户的余额。
相关推荐
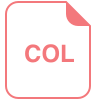
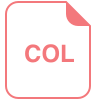
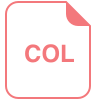
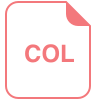
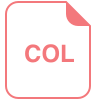












