帮我写一个贪吃蛇的html网页
时间: 2023-08-20 17:13:16 浏览: 52
好的,请参考下面的示例代码,这是一个简单的贪吃蛇游戏的 HTML 页面代码,使用了 HTML、CSS 和 JavaScript 技术:
```html
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇</title>
<style type="text/css">
body {
margin: 0;
padding: 0;
background-color: #EEE;
}
#game-board {
margin: 20px auto;
width: 400px;
height: 400px;
background-color: #FFF;
position: relative;
}
#game-board div {
position: absolute;
box-sizing: border-box;
border: 1px solid #DDD;
}
#snake-head {
background-color: #F00;
}
#snake-body {
background-color: #FF0;
}
#food {
background-color: #0F0;
}
</style>
</head>
<body>
<div id="game-board"></div>
<script type="text/javascript">
// 初始化游戏状态和画布
var gameBoard = document.getElementById('game-board');
var gridSize = 20; // 方格大小
var numRows = gameBoard.clientHeight / gridSize;
var numCols = gameBoard.clientWidth / gridSize;
var score = 0;
var snake = [{x: 5, y: 5}, {x: 4, y: 5}, {x: 3, y: 5}];
var direction = 'right';
var food = {x: Math.floor(Math.random() * numCols), y: Math.floor(Math.random() * numRows)};
// 绘制游戏画面
function drawGame() {
// 绘制蛇头和身体
for (var i = 0; i < snake.length; i++) {
var cell = document.createElement('div');
cell.style.width = gridSize + 'px';
cell.style.height = gridSize + 'px';
cell.style.top = snake[i].y * gridSize + 'px';
cell.style.left = snake[i].x * gridSize + 'px';
cell.id = (i == 0) ? 'snake-head' : 'snake-body';
gameBoard.appendChild(cell);
}
// 绘制食物
var cell = document.createElement('div');
cell.style.width = gridSize + 'px';
cell.style.height = gridSize + 'px';
cell.style.top = food.y * gridSize + 'px';
cell.style.left = food.x * gridSize + 'px';
cell.id = 'food';
gameBoard.appendChild(cell);
}
// 根据方向移动蛇的位置
function moveSnake() {
var head = snake[0];
var newHead = {x: head.x, y: head.y};
switch (direction) {
case 'up':
newHead.y -= 1;
break;
case 'down':
newHead.y += 1;
break;
case 'left':
newHead.x -= 1;
break;
case 'right':
newHead.x += 1;
break;
}
snake.unshift(newHead);
if (newHead.x == food.x && newHead.y == food.y) {
// 如果吃到了食物,则生成新的食物
score += 10;
food = {x: Math.floor(Math.random() * numCols), y: Math.floor(Math.random() * numRows)};
} else {
// 如果没有吃到食物,则移除蛇尾
snake.pop();
}
}
// 检测游戏是否结束
function checkGameOver() {
var head = snake[0];
if (head.x < 0 || head.x >= numCols || head.y < 0 || head.y >= numRows) {
return true; // 撞墙了
}
for (var i = 1; i < snake.length; i++) {
if (head.x == snake[i].x && head.y == snake[i].y) {
return true; // 撞到自己了
}
}
return false;
}
// 游戏循环
function gameLoop() {
// 移动蛇的位置
moveSnake();
// 检测游戏是否结束
if (checkGameOver()) {
alert('游戏结束,得分:' + score);
return;
}
// 清空游戏画面
gameBoard.innerHTML = '';
// 重新绘制游戏画面
drawGame();
// 延迟一段时间,再次运行游戏循环
setTimeout(gameLoop, 100);
}
// 监听键盘事件,改变蛇的方向
document.addEventListener('keydown', function(event) {
switch (event.keyCode) {
case 37: // 左箭头
if (direction != 'right') direction = 'left';
break;
case 38: // 上箭头
if (direction != 'down') direction = 'up';
break;
case 39: // 右箭头
if (direction != 'left') direction = 'right';
break;
case 40: // 下箭头
if (direction != 'up') direction = 'down';
break;
}
});
// 启动游戏循环
drawGame();
gameLoop();
</script>
</body>
</html>
```
这个示例代码实现了一个简单的贪吃蛇游戏,包括了游戏状态的维护、游戏画面的绘制、用户输入的处理和游戏逻辑的执行等功能。您可以在此基础上进行修改和扩展,实现更复杂和更有趣的贪吃蛇游戏。
阅读全文
相关推荐











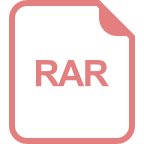

