C语言实现读取两个超大数存入链表并求和,不用考虑进位的情况并追加写在源文件中
时间: 2024-02-25 07:59:07 浏览: 76
好的,下面是一个简单的 C 代码示例,用于读取两个超大数并将它们存储在链表中,并计算它们的和,不考虑进位。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_DIGIT 1000000
typedef struct node {
int digit;
struct node *next;
} Node;
Node *create_node(int digit) {
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->digit = digit;
new_node->next = NULL;
return new_node;
}
Node *create_list(char *num) {
Node *head = NULL;
Node *tail = NULL;
int i = 0;
while (num[i] != '\0') {
int digit = num[i] - '0';
Node *new_node = create_node(digit);
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
i++;
}
return head;
}
void print_list(Node *head) {
Node *current = head;
while (current != NULL) {
printf("%d", current->digit);
current = current->next;
}
printf("\n");
}
Node *add_lists(Node *list1, Node *list2) {
Node *head = NULL;
Node *tail = NULL;
Node *current1 = list1;
Node *current2 = list2;
while (current1 != NULL && current2 != NULL) {
int sum = current1->digit + current2->digit;
Node *new_node = create_node(sum);
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
current1 = current1->next;
current2 = current2->next;
}
return head;
}
int main() {
char num1[MAX_DIGIT];
char num2[MAX_DIGIT];
printf("请输入两个超大数,以空格分隔:\n");
scanf("%s %s", num1, num2);
Node *list1 = create_list(num1);
Node *list2 = create_list(num2);
printf("链表1: ");
print_list(list1);
printf("链表2: ");
print_list(list2);
Node *sum_list = add_lists(list1, list2);
printf("和链表: ");
print_list(sum_list);
return 0;
}
```
在这个示例中,我们首先定义了一个节点结构,表示链表中的每个节点。然后,我们定义了一些函数来创建节点,创建链表,打印链表以及将两个链表相加。在主函数中,我们从用户输入中读取两个超大数,将它们存储在两个链表中,然后计算它们的和,并将和存储在一个新的链表中。
请注意,此示例仅处理不考虑进位的情况。在实际应用中,必须考虑进位的情况。
阅读全文
相关推荐
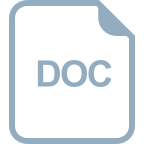
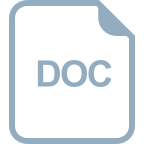
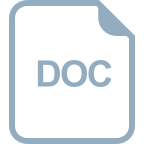
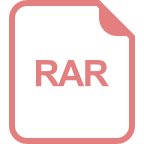
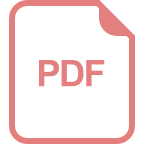
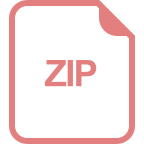
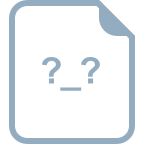
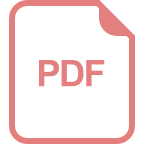
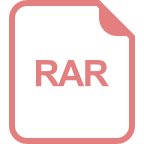
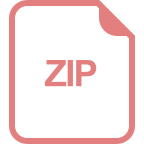
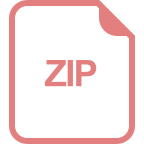
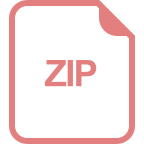
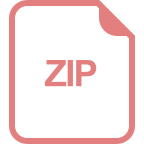
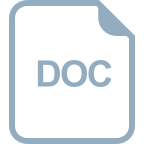
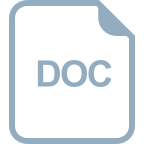
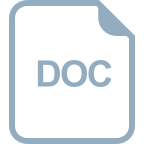