java登录界面代码加连接数据库
时间: 2023-09-11 09:12:15 浏览: 27
以下是一个简单的Java登录界面代码,包含连接MySQL数据库的实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.sql.*;
public class Login extends JFrame implements ActionListener {
private JLabel label1, label2;
private JTextField textField;
private JPasswordField passwordField;
private JButton button;
private Connection conn;
public Login() {
super("登录界面");
label1 = new JLabel("用户名:");
label2 = new JLabel("密码:");
textField = new JTextField(10);
passwordField = new JPasswordField(10);
button = new JButton("登录");
JPanel panel1 = new JPanel(new GridLayout(2,2));
panel1.add(label1);
panel1.add(textField);
panel1.add(label2);
panel1.add(passwordField);
JPanel panel2 = new JPanel(new FlowLayout());
panel2.add(button);
JPanel panel = new JPanel(new BorderLayout());
panel.add(panel1, BorderLayout.CENTER);
panel.add(panel2, BorderLayout.SOUTH);
add(panel);
button.addActionListener(this);
setSize(300, 150);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//连接数据库
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "root";
conn = DriverManager.getConnection(url, user, password);
} catch (SQLException | ClassNotFoundException e) {
e.printStackTrace();
}
}
@Override
public void actionPerformed(ActionEvent event) {
if (event.getSource() == button) {
String userName = textField.getText();
String password = new String(passwordField.getPassword());
try {
Statement stmt = conn.createStatement();
String sql = "SELECT * FROM user WHERE username='" + userName + "' AND password='" + password + "'";
ResultSet rs = stmt.executeQuery(sql);
if (rs.next()) {
JOptionPane.showMessageDialog(null, "登录成功");
} else {
JOptionPane.showMessageDialog(null, "用户名或密码错误");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
new Login();
}
}
```
上述代码中,我们使用了JDBC连接MySQL数据库,实现了用户输入用户名和密码后,从数据库中查询是否存在相应的用户。若存在,则弹出“登录成功”的消息框,否则弹出“用户名或密码错误”的消息框。
需要注意的是,在使用JDBC连接MySQL数据库时,需要先下载MySQL的JDBC驱动,然后将其添加到项目的classpath中。
相关推荐
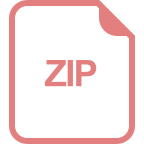
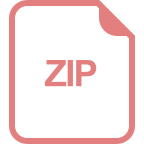















