如何用LSTM全连接层提取特征python代码
时间: 2023-03-29 11:03:16 浏览: 245
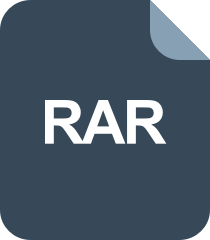
特征码提取

以下是使用 LSTM 全连接层提取特征的 Python 代码示例:
```python
from keras.models import Sequential
from keras.layers import LSTM, Dense
# 定义 LSTM 模型
model = Sequential()
model.add(LSTM(64, input_shape=(10, 32))) # 输入形状为 (batch_size, timesteps, input_dim)
model.add(Dense(16, activation='relu')) # 全连接层
model.summary()
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 生成随机数据
import numpy as np
x = np.random.randn(100, 10, 32)
y = np.random.randn(100, 16)
# 训练模型
model.fit(x, y, epochs=10, batch_size=32)
```
在上述代码中,我们使用 Keras 搭建了一个 LSTM 模型,其中包括一个 LSTM 层和一个全连接层。LSTM 层用于提取序列数据的特征,全连接层用于将 LSTM 层的输出转换为最终的预测结果。在训练模型时,我们可以使用随机生成的数据进行训练。
阅读全文
相关推荐
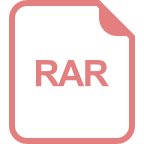
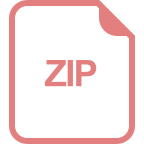

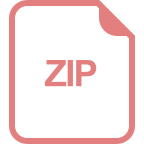
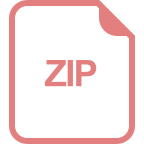












