package xjdz; import javax.swing.*; import java.awt.*; import java.io.IOException; import java.io.InputStream; public class GameWin extends JFrame { public GameWin() { initWindow(); } /** * 初始化窗口 */ private void initWindow() { this.setSize(999, 666); this.setTitle("oqcw星际大战仿飞机大战小游戏"); this.setResizable(false); this.setLocationRelativeTo(null); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 设置窗口背景 JLayeredPane layeredPane = new JLayeredPane(); setContentPane(layeredPane); // 添加背景图片 JLabel backgroundImageLabel = new JLabel(getBackgroundImageIcon()); backgroundImageLabel.setBounds(0, 0, getWidth(), getHeight()); layeredPane.add(backgroundImageLabel, new Integer(0)); } /** * 获取背景图片 */ private ImageIcon getBackgroundImageIcon() { try { InputStream is = this.getClass().getClassLoader().getResourceAsStream("bg.jpg"); byte[] bytes = new byte[is.available()]; is.read(bytes); is.close(); return new ImageIcon(bytes); } catch (IOException e) { e.printStackTrace(); return null; } } /** * 启动窗口 */ public void launch() { this.setVisible(true); } public static void main(String[] args) { GameWin gameWin = new GameWin(); gameWin.launch(); } /** * 程序退出时释放资源 */ @Override public void dispose() { super.dispose(); System.gc(); } }
时间: 2024-02-14 20:33:05 浏览: 72
这段代码是一个 Java Swing 窗口程序,实现了一个名为 "oqcw星际大战仿飞机大战小游戏" 的游戏窗口。在 initWindow() 方法中,设置了窗口的大小、标题、位置、不可调整大小和关闭操作。使用 JLayeredPane 添加了一个 JLabel 作为背景图片,并通过 getBackgroundImageIcon() 方法获取了背景图片的 ImageIcon。在 main() 方法中,创建了一个 GameWin 对象,并启动了窗口。dispose() 方法用于在程序退出时释放资源。
相关问题
package xjdz; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; public class GameWin extends JFrame{ public void launch(){ //launch启动 this.setVisible(true);//设置窗口是否可见 this.setSize(999, 666);//设置窗口大小 this.setLocationRelativeTo(null);//设置居中打开 this.setTitle("oqcw星际大战仿飞机大战小游戏");//设置窗口标题 this.setResizable(false);//设置游戏界面不可以改 this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置关闭窗口时退出程序 // this.setContentPane(new JLabel(new ImageIcon("src/bg.jpg")));//设置窗口背景 } public static void main(String[] args) { GameWin gameWin = new GameWin(); gameWin.launch(); } } 在此代码的基础上,加入一个登录注册面板
可以通过在 GameWin 类中添加一个 JPanel,来实现登录注册面板的功能。具体实现步骤如下:
1. 在 GameWin 类中添加一个 JPanel 变量,用于存放登录注册面板。
2. 在 launch 方法中,初始化登录注册面板,设置其大小和位置,并添加到窗口中。
3. 在登录注册面板中添加必要的组件,如文本框、标签和按钮等,用于实现登录和注册功能。
4. 根据需要,可以在登录注册面板中添加监听器,用于响应用户的操作。
下面是修改后的代码示例:
```java
package xjdz;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class GameWin extends JFrame{
private JPanel loginPanel; // 登录注册面板
public void launch(){
this.setVisible(true);
this.setSize(999, 666);
this.setLocationRelativeTo(null);
this.setTitle("oqcw星际大战仿飞机大战小游戏");
this.setResizable(false);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
// 初始化登录注册面板
loginPanel = new JPanel();
loginPanel.setLayout(null); // 使用绝对布局
loginPanel.setBounds(300, 200, 400, 200); // 设置大小和位置
this.getContentPane().add(loginPanel); // 添加到窗口中
// 在登录注册面板中添加组件
JLabel usernameLabel = new JLabel("用户名:");
usernameLabel.setBounds(50, 50, 60, 30);
loginPanel.add(usernameLabel);
JTextField usernameField = new JTextField();
usernameField.setBounds(120, 50, 200, 30);
loginPanel.add(usernameField);
JLabel passwordLabel = new JLabel("密码:");
passwordLabel.setBounds(50, 90, 60, 30);
loginPanel.add(passwordLabel);
JTextField passwordField = new JTextField();
passwordField.setBounds(120, 90, 200, 30);
loginPanel.add(passwordField);
JButton loginButton = new JButton("登录");
loginButton.setBounds(100, 130, 80, 30);
loginPanel.add(loginButton);
JButton registerButton = new JButton("注册");
registerButton.setBounds(220, 130, 80, 30);
loginPanel.add(registerButton);
}
public static void main(String[] args) {
GameWin gameWin = new GameWin();
gameWin.launch();
}
}
```
package xjdz; import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class GameWin extends JFrame{ public void launch(){ //launch启动 this.setVisible(true);//设置窗口是否可见 this.setSize(999, 666);//设置窗口大小 this.setLocationRelativeTo(null);//设置居中打开 this.setTitle("oqcw星际大战仿飞机大战小游戏");//设置窗口标题 this.setResizable(false);//设置游戏界面不可以改 this.setDefaultCloseOperation(EXIT_ON_CLOSE);//设置关闭窗口时退出程序 //设置窗口背景 JLayeredPane layeredPane = new JLayeredPane(); setContentPane(layeredPane); // Add a background image to the Layered Pane JLabel backgroundImageLabel = new JLabel(new ImageIcon("src/bg.jpg")); backgroundImageLabel.setBounds(0, 0, getWidth(), getHeight()); layeredPane.add(backgroundImageLabel, new Integer(0)); setVisible(true);
这段代码是一个Java Swing的窗口程序,创建了一个继承自JFrame的类GameWin,实现了launch方法来启动窗口。在launch方法中,设置了窗口的可见性、大小、居中打开、标题、不可改变大小、以及关闭窗口时退出程序。此外,还使用JLayeredPane来添加一个背景图片。最后通过setVisible方法设置窗口可见。
阅读全文
相关推荐
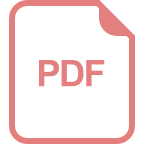





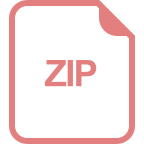
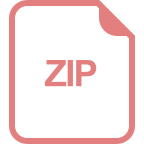
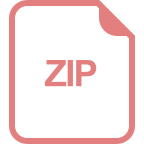
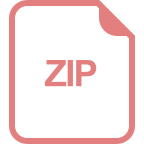
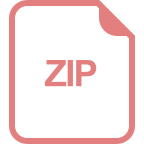