编写一个简单的学生信息管理程序及菜单系统。 1、定义学生类和管理员类。以学生类为基础,派生出本科生类和研究生类。学生包括学号、姓名、性别、年龄、专业、班级、成绩等成员;研究生还包括导师等信息,本科生还包括学制(4年或3年)等信息。 2、定义管理员类,然后编写一个登录界面,登录后才能进行各项操作;完成对学生信息进行管理,体现管理员类和学生类之间的组合关系,用链表组织学生信息。具体功能包括: (1) 学生信息显示:显示每个学生的所有信息; (2) 查找:可分别按学号、姓名、班级查询学生,并显示信息; (3) 删除:按学号或姓名删除某一学生; (4) 修改:对某一学生的信息进行修改; (5) 学生成绩汇总:求平均值,或某一分数段人数。 3、设计对应虚函数,构建多态体系,使得运行期能进行对象类型识别,实现研究生和本科生的统一管理。定义基类指针组成的数组链表,实现学生数据统一管理;在学生基类、本科生类、研究
时间: 2024-01-12 11:04:10 浏览: 23
生类中定义虚函数,实现多态。管理员类中可以定义一个基类指针数组,用于存储学生的信息,可以实现对学生信息的统一管理。
以下是一个简单的实现:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Student {
public:
string id;
string name;
string gender;
int age;
string major;
string clazz;
double score;
Student(string id, string name, string gender, int age, string major, string clazz, double score) {
this->id = id;
this->name = name;
this->gender = gender;
this->age = age;
this->major = major;
this->clazz = clazz;
this->score = score;
}
virtual void display() {
cout << "ID: " << id << endl;
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
cout << "Major: " << major << endl;
cout << "Class: " << clazz << endl;
cout << "Score: " << score << endl;
}
virtual double getScore() {
return score;
}
};
class Undergraduate : public Student {
public:
string studyTime;
Undergraduate(string id, string name, string gender, int age, string major, string clazz, double score, string studyTime)
: Student(id, name, gender, age, major, clazz, score) {
this->studyTime = studyTime;
}
virtual void display() {
Student::display();
cout << "Study Time: " << studyTime << endl;
}
};
class Graduate : public Student {
public:
string supervisor;
Graduate(string id, string name, string gender, int age, string major, string clazz, double score, string supervisor)
: Student(id, name, gender, age, major, clazz, score) {
this->supervisor = supervisor;
}
virtual void display() {
Student::display();
cout << "Supervisor: " << supervisor << endl;
}
};
class Admin {
private:
vector<Student *> students;
public:
void login() {
// 进行登录操作
}
void add(Student *student) {
students.push_back(student);
}
void displayAll() {
for (auto student : students) {
student->display();
cout << endl;
}
}
void findByID(string id) {
for (auto student : students) {
if (student->id == id) {
student->display();
return;
}
}
cout << "Student not found." << endl;
}
void findByName(string name) {
for (auto student : students) {
if (student->name == name) {
student->display();
return;
}
}
cout << "Student not found." << endl;
}
void findByClass(string clazz) {
for (auto student : students) {
if (student->clazz == clazz) {
student->display();
cout << endl;
}
}
}
void removeByID(string id) {
for (auto it = students.begin(); it != students.end(); ++it) {
if ((*it)->id == id) {
delete *it;
students.erase(it);
return;
}
}
cout << "Student not found." << endl;
}
void removeByName(string name) {
for (auto it = students.begin(); it != students.end(); ++it) {
if ((*it)->name == name) {
delete *it;
students.erase(it);
return;
}
}
cout << "Student not found." << endl;
}
void modifyByID(string id, string name, string gender, int age, string major, string clazz, double score) {
for (auto student : students) {
if (student->id == id) {
student->name = name;
student->gender = gender;
student->age = age;
student->major = major;
student->clazz = clazz;
student->score = score;
return;
}
}
cout << "Student not found." << endl;
}
void summary(double minScore, double maxScore) {
int count = 0;
double sum = 0;
for (auto student : students) {
double score = student->getScore();
if (score >= minScore && score <= maxScore) {
count++;
sum += score;
}
}
cout << "Count: " << count << endl;
cout << "Average score: " << (count == 0 ? 0 : sum / count) << endl;
}
};
int main() {
Admin admin;
admin.add(new Undergraduate("1001", "Alice", "Female", 20, "Computer Science", "Class 1", 90.0, "4 years"));
admin.add(new Undergraduate("1002", "Bob", "Male", 21, "Computer Science", "Class 2", 85.0, "4 years"));
admin.add(new Graduate("2001", "Charlie", "Male", 25, "Mathematics", "Class 3", 95.0, "Dr. Smith"));
admin.displayAll();
cout << endl;
admin.findByID("1001");
admin.findByID("1003");
cout << endl;
admin.findByName("Bob");
admin.findByName("David");
cout << endl;
admin.findByClass("Class 1");
cout << endl;
admin.removeByID("1002");
admin.displayAll();
cout << endl;
admin.modifyByID("1001", "Alice Smith", "Female", 20, "Computer Science", "Class 2", 95.0);
admin.findByID("1001");
cout << endl;
admin.summary(80.0, 90.0);
return 0;
}
```
该程序定义了一个学生类和两个派生类(本科生和研究生类),并且定义了一个管理员类用于管理学生信息。管理员类中包含了一些基本的操作,例如添加学生、显示所有学生信息、按学号或姓名查找学生、按学号或姓名删除学生、修改学生信息、以及计算学生成绩汇总等功能。程序中使用了虚函数实现了多态,使得可以对不同类型的学生进行统一管理。
相关推荐
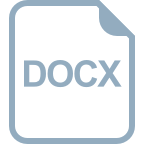
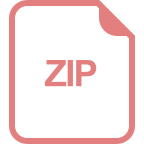
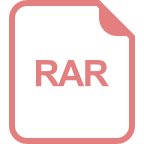















