def __init__
时间: 2023-10-08 21:13:37 浏览: 33
`__init__` 是 Python 中的一个特殊方法,用于对象的初始化。当一个类的实例被创建时,`__init__` 方法会自动被调用。
在 `__init__` 方法中,我们可以对对象的属性进行初始化,也可以执行其他的一些操作。例如:
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
person = Person("Alice", 25)
person.say_hello() # 输出 "Hello, my name is Alice and I am 25 years old."
```
在上面的例子中,`Person` 类有两个属性 `name` 和 `age`,在 `__init__` 方法中对这两个属性进行了初始化。在创建 `person` 对象时,传入了 `name` 和 `age` 参数,这些参数被用于初始化对象的属性。最后调用 `say_hello` 方法,输出对象的属性值。
相关问题
def __init__详解
`__init__`是Python类中的一个特殊方法,用于在创建对象时进行初始化操作。每当创建一个对象时,`__init__`方法会被自动调用,从而可以对对象进行初始化操作,并为对象设置属性和方法。
`__init__`方法的语法如下:
```python
class MyClass:
def __init__(self, arg1, arg2, ...):
# initialization statements
```
其中,`self`表示当前对象本身,`arg1`、`arg2`等表示初始化时传入的参数。在`__init__`方法中,可以对对象进行各种初始化操作,例如设置属性、调用其他方法等。
下面是一个示例,展示了`__init__`方法的使用:
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name}, and I am {self.age} years old.")
person1 = Person("Alice", 25)
person2 = Person("Bob", 30)
person1.say_hello() # 输出:Hello, my name is Alice, and I am 25 years old.
person2.say_hello() # 输出:Hello, my name is Bob, and I am 30 years old.
```
在上面的示例中,我们定义了一个`Person`类,该类有两个属性`name`和`age`,并且有一个`say_hello`方法,用于打印出自我介绍的信息。在初始化时,我们传入了`name`和`age`两个参数,并将它们分别赋值给对象的`name`和`age`属性。然后我们创建了两个`Person`对象,分别为`person1`和`person2`,并分别调用了它们的`say_hello`方法,输出了它们的自我介绍信息。
python def __init__
在Python中,`def __init__`是一个特殊的方法,用于在使用类创建对象之后进行初始化操作。这个方法会在对象创建时自动执行,并且可以用来给新创建的对象初始化属性。通常,我们会在`__init__`方法中使用`self`关键字来引用对象自身,并通过参数来传递属性的初始值。例如,`def __init__(self, name, age):`表示定义了一个`__init__`方法,该方法接受两个参数`name`和`age`,并将它们分别赋值给对象的`name`和`age`属性。这样,在创建对象时,我们可以通过传递参数来初始化对象的属性。例如,`p = Person('杰拉考', 200)`就是创建了一个名为`p`的`Person`对象,并将`name`属性设置为`'杰拉考'`,`age`属性设置为`200`。通过使用点`.`运算符,我们可以访问对象的属性,例如`print(p.name)`和`print(p.age)`可以分别输出对象`p`的名字和年龄。\[1\]\[2\]
#### 引用[.reference_title]
- *1* *2* *3* [Python中 __init__的通俗解释](https://blog.csdn.net/tlammon/article/details/105449361)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
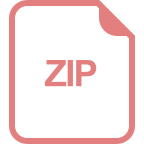
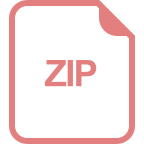
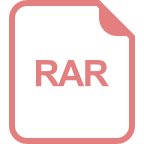







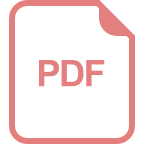
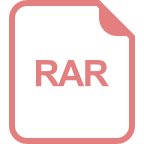
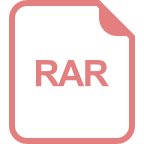
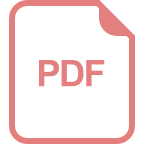
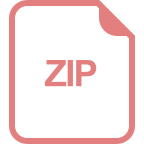
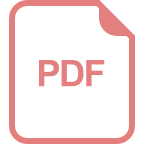
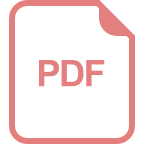