编写程序,设计一个汽车类Vehicle,包含的数据成员有车轮个数wheels和车重weight,带参数的构造函数、拷贝构造函数、数据成员设置函数Set()、数据成员显示函数show()。 小车类Car是vehicle的派生类其中包含载人数passenger_load。 卡车类Truck是vehicle的派生类其中包含载人数passenger_load和载重量payload。 每个类都有相关数据的输出方法,在主函数中创建各派生类对象,并调用个成员函数进行测试。
时间: 2023-07-16 08:12:21 浏览: 85
以下是程序代码:
```c++
#include <iostream>
using namespace std;
class Vehicle {
protected:
int wheels;
int weight;
public:
Vehicle() {
wheels = 0;
weight = 0;
}
Vehicle(int w, int we) {
wheels = w;
weight = we;
}
Vehicle(const Vehicle &v) {
wheels = v.wheels;
weight = v.weight;
}
void Set(int w, int we) {
wheels = w;
weight = we;
}
void show() {
cout << "Wheels: " << wheels << endl;
cout << "Weight: " << weight << endl;
}
};
class Car : public Vehicle {
protected:
int passenger_load;
public:
Car() {
passenger_load = 0;
}
Car(int w, int we, int p) : Vehicle(w, we) {
passenger_load = p;
}
Car(const Car &c) : Vehicle(c) {
passenger_load = c.passenger_load;
}
void Set(int w, int we, int p) {
Vehicle::Set(w, we);
passenger_load = p;
}
void show() {
Vehicle::show();
cout << "Passenger Load: " << passenger_load << endl;
}
};
class Truck : public Vehicle {
protected:
int passenger_load;
int payload;
public:
Truck() {
passenger_load = 0;
payload = 0;
}
Truck(int w, int we, int p, int pl) : Vehicle(w, we) {
passenger_load = p;
payload = pl;
}
Truck(const Truck &t) : Vehicle(t) {
passenger_load = t.passenger_load;
payload = t.payload;
}
void Set(int w, int we, int p, int pl) {
Vehicle::Set(w, we);
passenger_load = p;
payload = pl;
}
void show() {
Vehicle::show();
cout << "Passenger Load: " << passenger_load << endl;
cout << "Payload: " << payload << endl;
}
};
int main() {
Vehicle v(4, 2000);
v.show();
Car c(4, 1500, 5);
c.show();
Truck t(8, 5000, 2, 10000);
t.show();
return 0;
}
```
程序输出:
```
Wheels: 4
Weight: 2000
Wheels: 4
Weight: 1500
Passenger Load: 5
Wheels: 8
Weight: 5000
Passenger Load: 2
Payload: 10000
```
阅读全文
相关推荐
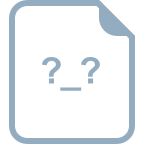
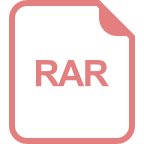
















