在上一个代码中加入在矩形中间画一个点并输出矩形中间坐标的功能
时间: 2024-04-09 15:30:00 浏览: 98
你可以在上面的代码中加入一个步骤来画一个点,并输出矩形中心的坐标。下面是修改后的代码示例:
```python
import cv2
import numpy as np
def match_pattern(frame, pattern):
# 载入模式图像
pattern_img = cv2.imread(pattern)
# 获取模式图像的宽高
pattern_height, pattern_width, _ = pattern_img.shape
# 使用模板匹配算法进行匹配
result = cv2.matchTemplate(frame, pattern_img, cv2.TM_CCOEFF_NORMED)
# 设置匹配阈值
threshold = 0.8
# 寻找匹配结果中大于阈值的坐标
locations = np.where(result >= threshold)
for loc in zip(*locations[::-1]):
# 在原始图像上绘制矩形框标识匹配位置
cv2.rectangle(frame, loc, (loc[0] + pattern_width, loc[1] + pattern_height), (0, 255, 0), 2)
# 计算矩形中心坐标
center_x = loc[0] + pattern_width // 2
center_y = loc[1] + pattern_height // 2
# 在矩形中心画一个点
cv2.circle(frame, (center_x, center_y), 3, (255, 0, 0), -1)
# 输出矩形中心坐标
print("矩形中心坐标:({}, {})".format(center_x, center_y))
# 返回绘制了矩形框的图像
return frame
cap = cv2.VideoCapture(0)
# 设置模式图像的文件名
pattern_file = 'pattern.png'
while True:
ret, frame = cap.read()
# 调用函数进行模式匹配
result_frame = match_pattern(frame, pattern_file)
# 显示结果图像
cv2.imshow('Pattern Matching Result', result_frame)
# 如果按下q键则退出循环
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头资源和关闭窗口
cap.release()
cv2.destroyAllWindows()
```
在这个修改后的代码中,我们在绘制矩形框之后计算矩形中心的坐标,并使用cv2.circle函数在矩形中心画一个蓝色的点。然后,我们通过print语句输出矩形中心的坐标。这样,每次找到匹配位置时,都会输出矩形中心的坐标。
希望这个修改后的代码对你有帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
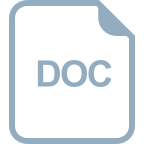
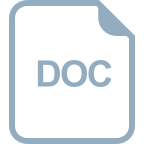
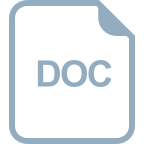
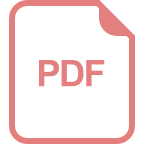
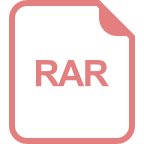
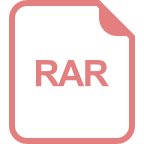
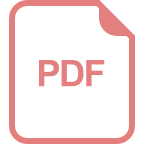
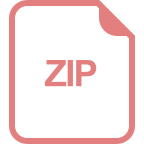
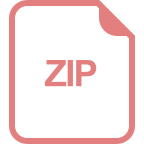
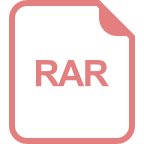
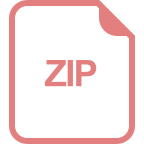
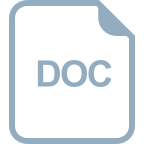
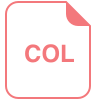
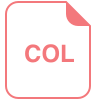
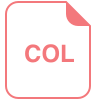
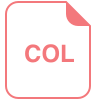
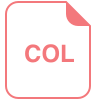

