html 鼠标移上去显示x号
时间: 2023-08-15 11:07:29 浏览: 88
可以使用CSS来实现这个效果。具体做法如下:
1. 在HTML中添加一个需要显示x号的元素,比如一个div或者span标签。
2. 在CSS中为该元素添加:hover伪类选择器,并设置其content属性为"x"。
例如,以下是一个div元素在鼠标移上去时显示x号的代码:
HTML:
```
<div class="hover-div">鼠标移上来试试</div>
```
CSS:
```
.hover-div:hover::before {
content: "x";
color: red;
}
```
这里使用了::before伪元素来在div元素前面添加x号,并设置了其颜色为红色。当鼠标移动到div元素上时,就会显示x号。
相关问题
html鼠标移到图片不同位置显示不同图片
可以使用HTML和JavaScript来实现鼠标移到图片不同位置显示不同图片的效果,具体实现方法如下:
1. 在HTML文件中,使用`<img>`标签插入需要显示的图片,并设置其id属性,例如:
```
<img src="image.jpg" id="myImage">
```
2. 在JavaScript中,使用`addEventListener()`方法为该图片添加鼠标移动事件,例如:
```
document.getElementById("myImage").addEventListener("mousemove", showImage);
function showImage(event) {
// 获取鼠标在图片上的位置
var x = event.clientX;
var y = event.clientY;
// 根据位置判断应该显示哪张图片
var imageSrc = "";
if (x < 100 && y < 100) {
imageSrc = "image1.jpg";
} else if (x < 200 && y < 200) {
imageSrc = "image2.jpg";
} else {
imageSrc = "image3.jpg";
}
// 更新图片的src属性
document.getElementById("myImage").src = imageSrc;
}
```
3. 在CSS中,为该图片设置一定的宽度和高度,以确保鼠标移动事件能够触发。
需要注意的是,该方法需要确保图片的位置和大小已经确定,否则可能会出现位置判断错误的问题。
idea springboot工程 如何鼠标移上去显示 i18n国际化的内容
在 Spring Boot 工程中,要实现鼠标移上去显示 i18n 国际化的内容,可以采用以下步骤:
1. 在 Spring Boot 工程中添加支持 i18n 的相关依赖,如 `spring-boot-starter-validation` 和 `spring-boot-starter-internationalization`。
2. 在 `application.properties` 或 `application.yml` 中配置 i18n 相关配置,如语言列表和资源文件位置等信息。
3. 在需要显示多语言文本的地方,使用 `MessageSource` 来获取对应语言的文本。
4. 使用 Thymeleaf 模板引擎来渲染页面,并在页面中使用 Thymeleaf 提供的 `th:attr` 属性和 `data-*` 属性来实现鼠标移入显示多语言文本的效果。
示例代码如下:
1. 配置文件
```yaml
spring:
messages:
basename: i18n/messages
```
2. 控制器
```java
@Controller
public class MyController {
@Autowired
private MessageSource messageSource;
@GetMapping("/")
public String index(Model model, Locale locale) {
String hello = messageSource.getMessage("hello", null, locale);
String world = messageSource.getMessage("world", null, locale);
model.addAttribute("hello", hello);
model.addAttribute("world", world);
return "index";
}
}
```
3. 页面
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>My Page</title>
<style>
.tooltip {
position: absolute;
display: none;
border: 1px solid #ccc;
background-color: #fff;
padding: 5px;
}
</style>
</head>
<body>
<h1 th:text="${hello}"></h1>
<p th:text="${world}" th:attr="data-i18n='world'"></p>
<script>
function showTooltip(event) {
var lang = thymeleafFragmentParameters.locale;
var key = event.target.getAttribute('data-i18n');
var text = /* 使用ajax异步获取对应的文本 */;
var tooltip = document.createElement('div');
tooltip.className = 'tooltip';
tooltip.textContent = text;
document.body.appendChild(tooltip);
var x = event.pageX;
var y = event.pageY;
tooltip.style.left = x + 'px';
tooltip.style.top = y + 'px';
event.target.addEventListener('mouseleave', function() {
document.body.removeChild(tooltip);
});
}
var elements = document.querySelectorAll('[data-i18n]');
for (var i = 0; i < elements.length; i++) {
elements[i].addEventListener('mouseenter', showTooltip);
}
</script>
</body>
</html>
```
在上面的示例代码中,我们在 `application.yml` 中配置了 i18n 相关配置,使用 `MessageSource` 来获取对应语言的文本,使用 Thymeleaf 模板引擎来渲染页面,并在页面中使用 Thymeleaf 提供的 `th:attr` 属性和 `data-*` 属性来实现鼠标移入显示多语言文本的效果。同时,我们使用了 JavaScript 代码来异步获取多语言文本,并在页面上添加对应的提示框。
相关推荐
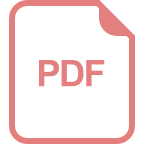
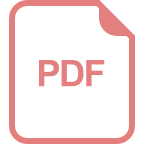
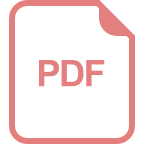
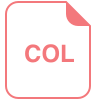
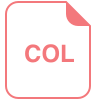
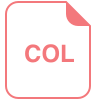
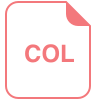
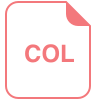







