iDEA验证码代码怎么写
时间: 2024-01-22 18:49:12 浏览: 19
iDEA验证码是一种图形验证码,可以通过以下步骤进行编写:
1. 生成随机字符串,可以使用 Python 的 random 模块或者其他语言的随机数函数。
2. 将随机字符串进行加密,使用 iDEA 算法进行加密。
3. 将加密后的字符串生成图片,可以使用 Python 的 PIL 或者其他语言的图像处理库。
4. 将生成的图片返回给用户,用户需要输入正确的验证码才能进行下一步操作。
以下是 Python 的示例代码:
```python
from PIL import Image, ImageDraw, ImageFont
import random
# 生成随机字符串
def generate_random_string(length=4):
chars = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890'
return ''.join(random.sample(chars, length))
# IDEA加密算法
def encrypt(data):
# TODO: 实现 IDEA 算法加密
# 生成验证码图片
def generate_captcha_image(text):
# 设置图片大小
width, height = 100, 40
# 创建图片对象
image = Image.new('RGB', (width, height), (255, 255, 255))
# 创建画笔对象
draw = ImageDraw.Draw(image)
# 设置字体
font = ImageFont.truetype('arial.ttf', 30)
# 在图片上写入文字
draw.text((10, 5), text, font=font, fill=(0, 0, 0))
# 生成干扰点
for i in range(100):
x = random.randint(0, width)
y = random.randint(0, height)
draw.point((x, y), fill=(0, 0, 0))
# 生成干扰线
for i in range(5):
x1 = random.randint(0, width)
y1 = random.randint(0, height)
x2 = random.randint(0, width)
y2 = random.randint(0, height)
draw.line((x1, y1, x2, y2), fill=(0, 0, 0), width=2)
# 返回图片对象
return image
# 生成验证码
def generate_captcha():
# 生成随机字符串
text = generate_random_string()
# IDEA加密算法
encrypted_text = encrypt(text)
# 生成验证码图片
image = generate_captcha_image(text)
# 返回验证码图片和加密后的字符串
return image, encrypted_text
```
注意:以上代码中的 IDEA 加密算法部分需要自行实现。
相关推荐












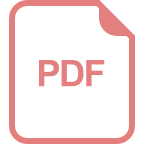