java取出数据库blob格式文件
时间: 2023-07-08 18:26:44 浏览: 70
在 Java 中,使用 JDBC 可以从数据库中读取 BLOB 格式的数据。具体步骤如下:
1. 使用 `java.sql.Connection` 接口获取数据库连接。
```java
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "username", "password");
```
2. 创建 `java.sql.PreparedStatement` 对象,并执行查询。
```java
PreparedStatement pstmt = conn.prepareStatement("SELECT blob_data FROM my_table WHERE id = ?");
pstmt.setInt(1, 1);
ResultSet rs = pstmt.executeQuery();
```
这里假设我们要查询表 `my_table` 中 `id` 为 1 的记录的 `blob_data` 字段。
3. 从结果集中获取 BLOB 类型的数据,并将其保存到文件中。
```java
if (rs.next()) {
Blob blob = rs.getBlob("blob_data"); // 获取 BLOB 类型的数据
InputStream in = blob.getBinaryStream(); // 获取 BLOB 类型数据的二进制流
OutputStream out = new FileOutputStream("output-file.dat"); // 创建输出流
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead); // 将数据写入输出流
}
out.close();
in.close();
}
```
在这个示例中,我们从结果集中获取 BLOB 类型的数据,然后使用 `java.io.InputStream` 将其读取为二进制流,并使用 `java.io.OutputStream` 将其写入到文件中。最后,记得关闭输入输出流。
完整代码示例:
```java
import java.io.*;
import java.sql.*;
public class ReadBlobExample {
public static void main(String[] args) {
try {
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "username", "password");
PreparedStatement pstmt = conn.prepareStatement("SELECT blob_data FROM my_table WHERE id = ?");
pstmt.setInt(1, 1);
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
Blob blob = rs.getBlob("blob_data");
InputStream in = blob.getBinaryStream();
OutputStream out = new FileOutputStream("output-file.dat");
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
out.close();
in.close();
}
rs.close();
pstmt.close();
conn.close();
} catch (SQLException ex) {
ex.printStackTrace();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
```
相关推荐
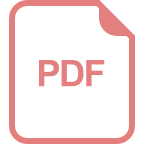













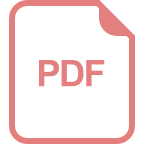
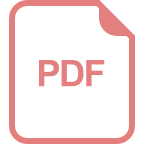