public void test() throws Exception {//将图片存到数据库 File file = new File("D:\\pictureTest\\new.doc"); FileInputStream fis = new FileInputStream(file); ByteArrayOutputStream baos = new ByteArrayOutputStream(); int len = -1; byte[] buf = new byte[1024]; while((len = fis.read(buf)) != -1) {//汇总字节流到内存 baos.write(buf, 0, len); } baos.close(); fis.close(); byte[] bytes = baos.toByteArray();//从内存取出字节流数组 Blob pic = conn.createBlob(); pic.setBytes(1, bytes);//把字节流设置给blob类 String sql = "insert into images(image) values (?)"; PreparedStatement ppst = conn.prepareStatement(sql); ppst.setBlob(1, pic); ppst.execute(); ppst.close(); }
时间: 2024-02-15 10:27:32 浏览: 23
这段代码使用Java中的IO操作将一个文件存储到数据库中。具体实现步骤如下:
1. 创建一个文件输入流FileInputStream,用于读取指定文件D:\\pictureTest\\new.doc中的数据。
2. 创建一个ByteArrayOutputStream,用于将读取到的数据以字节数组的形式写入内存缓冲区中,并支持动态扩容。
3. 创建一个缓冲区byte[],用于存储每次读取到的数据。
4. 循环读取文件输入流中的数据,将每次读取到的数据写入ByteArrayOutputStream中。
5. 关闭文件输入流和ByteArrayOutputStream,释放资源。
6. 从ByteArrayOutputStream中获取字节数组byte[],用于存储到数据库中。
7. 创建一个Blob对象,用于存储字节数组。
8. 将字节数组设置给Blob对象。
9. 创建一个PreparedStatement对象,用于执行SQL语句。
10. 将Blob对象设置给PreparedStatement对象中的占位符。
11. 执行SQL语句。
12. 关闭PreparedStatement对象,释放资源。
需要注意的是,这段代码中使用了文件输入流和ByteArrayOutputStream,需要及时关闭这两个流并释放资源,避免内存泄漏。同时,如果存储的文件较大,可能会导致内存溢出问题,需要特别注意。
相关问题
public static void main(String[] args) throws IOException { File file = new File("D:/360Downloads/tupian.jpg"); BufferedImage image = ImageIO.read(file); LumAdjust lumAdjust = new LumAdjust(); lumAdjust.lumAdjustment(image, 10); File file2 = new File("D:/360Downloads/2.jpg"); file2.createNewFile(); ImageIO.write(image, "JPG", file2);
这段 Java 代码的作用是读取一张图片,调整其亮度,并将调整后的图片保存到另一个文件中。
具体来说,代码的第1行声明了一个 `main` 方法,第2行创建一个 `File` 对象,用于指定要读取的图片文件路径。第3行使用 `ImageIO` 类中的 `read` 方法读取指定路径的图片文件,并将其读取到 `BufferedImage` 对象中。
接下来,在第4行中创建了一个 `LumAdjust` 对象,这是一个自定义的类,用于调整图片的亮度。第5行调用 `lumAdjustment` 方法,将读取到的图片和亮度调整参数(10)作为参数传入。这个方法会对图片进行亮度调整操作。
第6行创建了一个新的 `File` 对象,用于指定要保存的图片文件路径。第7行调用 `createNewFile` 方法创建一个新的空文件。第8行使用 `ImageIO` 类中的 `write` 方法将亮度调整后的图片保存到指定路径的文件中,文件格式为 JPG。
需要注意的是,这段代码可能会抛出 `IOException` 异常,因此在方法头部需要使用 `throws` 关键字声明抛出异常。
import java.io.*; public class Demo { public static void main(String[] args) throws Exception { File fos=new File("C:/动漫台词.txt"); fos.mkdirs(); FileOutputStream fos1=new FileOutputStream("C:/火影忍者.txt"); fos1.write("我会证明就算没有才华
,也可以成为一名优秀的忍者!".getBytes()); FileInputStream fis=new FileInputStream("C:/火影忍者.txt"); byte[] b=new byte[1024]; int len=fis.read(b); System.out.println(new String(b,0,len)); fis.close(); fos1.close(); } }
这段代码的作用是什么?
相关推荐
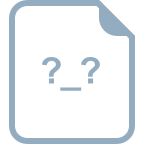
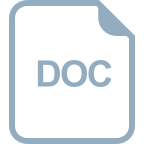
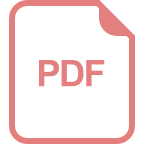













