c语言编程怎么调用api,怎么使用C语言编程读取从电脑usb接口的信息。
时间: 2024-02-12 13:05:12 浏览: 128
要调用API,首先需要包含相应的头文件,然后使用相应的函数。例如,要调用Windows API中的MessageBox函数,需要包含Windows.h头文件,然后使用MessageBox函数,如下所示:
```c
#include <Windows.h>
int main() {
MessageBox(NULL, "Hello, World!", "Message", MB_OK);
return 0;
}
```
要使用C语言编程读取从电脑USB接口的信息,需要使用USB通信协议,并调用相应的API函数。通常情况下,USB设备需要驱动程序才能被操作系统识别和使用。对于Windows操作系统,可以使用WinUSB API来与USB设备进行通信。具体步骤如下:
1. 使用SetupDiGetClassDevs函数获取指定设备类的设备信息列表。
2. 使用SetupDiEnumDeviceInterfaces函数获取设备的接口信息。
3. 使用SetupDiGetDeviceInterfaceDetail函数获取设备接口的详细信息。
4. 使用CreateFile函数打开设备。
5. 使用ReadFile和WriteFile函数读写设备数据。
6. 使用CloseHandle函数关闭设备。
下面是一个简单的例子,演示如何使用WinUSB API从USB设备中读取数据:
```c
#include <windows.h>
#include <SetupAPI.h>
#include <winusb.h>
#define VID 0x1234
#define PID 0x5678
int main() {
GUID guid;
HDEVINFO deviceInfoSet;
SP_DEVICE_INTERFACE_DATA deviceInterfaceData;
PSP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData;
HANDLE deviceHandle;
WINUSB_INTERFACE_HANDLE winusbHandle;
UCHAR buffer[1024];
DWORD bytesRead;
BOOL result;
// 初始化GUID
result = WinUsb_Initialize(VID, PID, &guid);
if (!result) {
printf("Failed to initialize GUID.\n");
return 1;
}
// 获取设备信息列表
deviceInfoSet = SetupDiGetClassDevs(&guid, NULL, NULL, DIGCF_PRESENT | DIGCF_DEVICEINTERFACE);
if (deviceInfoSet == INVALID_HANDLE_VALUE) {
printf("Failed to get device information set.\n");
return 1;
}
// 获取设备接口信息
deviceInterfaceData.cbSize = sizeof(SP_DEVICE_INTERFACE_DATA);
result = SetupDiEnumDeviceInterfaces(deviceInfoSet, NULL, &guid, 0, &deviceInterfaceData);
if (!result) {
printf("Failed to enumerate device interfaces.\n");
return 1;
}
// 获取设备接口的详细信息
DWORD size;
result = SetupDiGetDeviceInterfaceDetail(deviceInfoSet, &deviceInterfaceData, NULL, 0, &size, NULL);
if (!result && GetLastError() != ERROR_INSUFFICIENT_BUFFER) {
printf("Failed to get device interface detail size.\n");
return 1;
}
deviceInterfaceDetailData = (PSP_DEVICE_INTERFACE_DETAIL_DATA)malloc(size);
deviceInterfaceDetailData->cbSize = sizeof(SP_DEVICE_INTERFACE_DETAIL_DATA);
result = SetupDiGetDeviceInterfaceDetail(deviceInfoSet, &deviceInterfaceData, deviceInterfaceDetailData, size, NULL, NULL);
if (!result) {
printf("Failed to get device interface detail.\n");
return 1;
}
// 打开设备
deviceHandle = CreateFile(deviceInterfaceDetailData->DevicePath, GENERIC_READ | GENERIC_WRITE, FILE_SHARE_READ | FILE_SHARE_WRITE, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (deviceHandle == INVALID_HANDLE_VALUE) {
printf("Failed to open device.\n");
return 1;
}
// 初始化WinUSB
result = WinUsb_Initialize(deviceHandle, &winusbHandle);
if (!result) {
printf("Failed to initialize WinUSB.\n");
CloseHandle(deviceHandle);
return 1;
}
// 读取数据
result = WinUsb_ReadPipe(winusbHandle, 0x81, buffer, sizeof(buffer), &bytesRead, NULL);
if (!result) {
printf("Failed to read pipe.\n");
WinUsb_Free(winusbHandle);
CloseHandle(deviceHandle);
return 1;
}
// 关闭设备
WinUsb_Free(winusbHandle);
CloseHandle(deviceHandle);
return 0;
}
```
需要注意的是,上面的代码只是一个简单的例子,实际上与USB设备通信需要考虑很多问题,如设备的连接和断开、数据包的解析和组装等等。
阅读全文
相关推荐



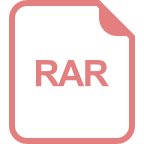












