写一段使用qt实现图像中特征点检测与匹配的代码
时间: 2024-05-12 07:16:08 浏览: 181
当然,我可以为您提供代码。以下是使用Qt实现图像中特征点检测与匹配的示例代码(使用OpenCV库):
```cpp
#include <opencv2/opencv.hpp>
#include <QDebug>
#include <QString>
#include <QImage>
#include <QPixmap>
#include <QHBoxLayout>
#include <QVBoxLayout>
#include <QLabel>
#include <QFileDialog>
#include <QPushButton>
#include <QCoreApplication>
#include "mainwindow.h"
#include "ui_mainwindow.h"
using namespace cv;
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
ui->matchButton->setEnabled(false); // 初始化时“匹配”按钮不可用
ui->feature1Label->setAlignment(Qt::AlignCenter); // 特征点图像居中显示
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::on_loadButton_clicked()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("选择图像"), QDir::homePath(), tr("Image Files (*.png *.jpg *.bmp)"));
if(fileName.isNull())
{
return;
}
// 读取图像
Mat img = imread(fileName.toStdString(), IMREAD_GRAYSCALE);
if(img.empty())
{
qWarning() << "读取图像失败:" << fileName;
return;
}
// 显示图像
QImage qimg = QImage(img.data, img.cols, img.rows, img.step, QImage::Format_Grayscale8);
QPixmap pixmap = QPixmap::fromImage(qimg);
ui->originalLabel->setPixmap(pixmap.scaled(ui->originalLabel->size(), Qt::KeepAspectRatio));
// 检测特征点
Ptr<FeatureDetector> detector = ORB::create();
std::vector<KeyPoint> keyPoints;
detector->detect(img, keyPoints);
// 在图像中标出特征点位置
cv::Mat imgKeypoints;
cv::drawKeypoints(img, keyPoints, imgKeypoints);
QImage qimgKeypoints = QImage(imgKeypoints.data, imgKeypoints.cols, imgKeypoints.rows, imgKeypoints.step, QImage::Format_Grayscale8);
QPixmap pixmapKeypoints = QPixmap::fromImage(qimgKeypoints);
ui->feature1Label->setPixmap(pixmapKeypoints.scaled(ui->feature1Label->size(), Qt::KeepAspectRatio));
// 保存特征点
this->keyPoints1 = keyPoints;
ui->matchButton->setEnabled(keyPoints.size() > 0);
}
void MainWindow::on_matchButton_clicked()
{
QString fileName = QFileDialog::getOpenFileName(this, tr("选择图像"), QDir::homePath(), tr("Image Files (*.png *.jpg *.bmp)"));
if(fileName.isNull())
{
return;
}
// 读取图像
Mat img = imread(fileName.toStdString(), IMREAD_GRAYSCALE);
if(img.empty())
{
qWarning() << "读取图像失败:" << fileName;
return;
}
// 显示图像
QImage qimg = QImage(img.data, img.cols, img.rows, img.step, QImage::Format_Grayscale8);
QPixmap pixmap = QPixmap::fromImage(qimg);
ui->matchingLabel->setPixmap(pixmap.scaled(ui->matchingLabel->size(), Qt::KeepAspectRatio));
// 检测特征点
Ptr<FeatureDetector> detector = ORB::create();
std::vector<KeyPoint> keyPoints;
detector->detect(img, keyPoints);
// 计算描述符
Ptr<DescriptorExtractor> descriptor = ORB::create();
cv::Mat descriptors;
descriptor->compute(img, keyPoints, descriptors);
// 匹配特征点
cv::BFMatcher matcher(cv::NORM_HAMMING2, false);
std::vector<cv::DMatch> matches;
if(this->keyPoints1.size() > 0)
{
matcher.match(this->descriptors1, descriptors, matches);
}
// 在图像中标出匹配的特征点位置
cv::Mat imgMatches;
cv::drawMatches(this->originalImg, this->keyPoints1, img, keyPoints, matches, imgMatches);
QImage qimgMatches = QImage(imgMatches.data, imgMatches.cols, imgMatches.rows, imgMatches.step, QImage::Format_Grayscale8);
QPixmap pixmapMatches = QPixmap::fromImage(qimgMatches);
ui->matchingLabel->setPixmap(pixmapMatches.scaled(ui->matchingLabel->size(), Qt::KeepAspectRatio));
// 显示匹配结果信息
QString matchInfo = QString("匹配到 %1 个特征点").arg(matches.size());
ui->matchInfoLabel->setText(matchInfo);
}
```
以上是Qt实现图像中特征点检测与匹配的示例代码,希望能对您有所帮助!
阅读全文
相关推荐
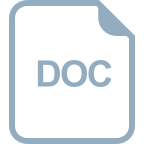
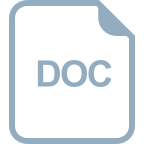
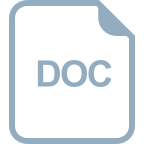
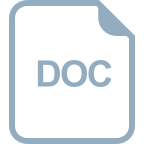
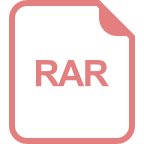
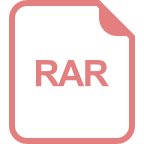
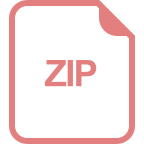
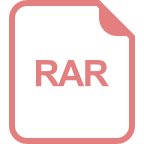
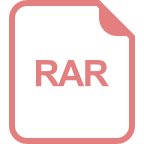
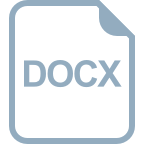
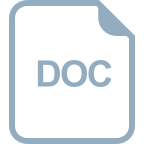
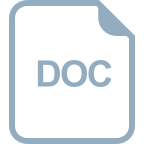
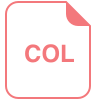
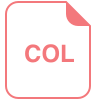
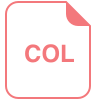
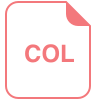
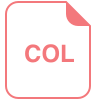

