import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; public class TreeBuilder { public static List<Node> buildTree(List<Node> nodes) { Map<Integer, Node> nodeMap = new HashMap<>(); List<Node> rootNodes = new ArrayList<>(); // 将节点放入map中,方便查找 for (Node node : nodes) { nodeMap.put(node.getId(), node); } // 遍历节点,将每个节点挂在其父节点下 for (Node node : nodes) { Node parent = nodeMap.get(node.getParentId()); if (parent != null) { parent.addChild(node); } else { rootNodes.add(node); } } return rootNodes; } } class Node { private int id; private int parentId; private List<Node> children = new ArrayList<>(); public Node(int id, int parentId) { this.id = id; this.parentId = parentId; } public int getId() { return id; } public int getParentId() { return parentId; } public List<Node> getChildren() { return children; } public void addChild(Node child) { children.add(child); } } 这一段代码没有把节点按照父子节点的关系层层组装返回给我
时间: 2024-02-14 08:13:44 浏览: 19
这段代码实现了将一个列表中的节点按照父子关系组装成一棵树,并返回根节点列表。如果你想要得到一棵组装好的树,你需要调用 `TreeBuilder.buildTree()` 方法。例如:
```
List<Node> nodes = new ArrayList<>();
// 添加节点到 nodes 列表中
List<Node> rootNodes = TreeBuilder.buildTree(nodes);
```
其中,`rootNodes` 就是组装好的根节点列表。你可以遍历 `rootNodes` 中的每一个节点,通过 `Node.getChildren()` 方法获取其子节点列表,以此类推,就可以遍历整棵树了。
相关问题
import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map;
这是一组 import 语句,用于导入 Java 标准库中的 ArrayList、HashMap、List 和 Map 类。在 Java 中,如果要使用某个类或接口,需要先通过 import 语句将其引入到当前代码文件中,才能在代码中使用。其中 ArrayList 是一个可变长度的数组实现类,HashMap 是一个哈希表实现类,List 是一个接口,定义了列表数据结构的基本操作,Map 是一个映射表接口,定义了键值对的基本操作。通过使用这些类和接口,可以方便地实现各种数据结构和算法。
import java.util.ArrayList;可以用其他语句替换吗
可以,这句话是导入Java中的ArrayList类,如果你不需要使用ArrayList类,可以将其替换为其他需要导入的类的语句。例如,如果你需要使用Java中的HashMap类,可以使用以下语句进行导入:
```
import java.util.HashMap;
```
相关推荐
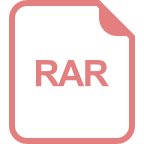
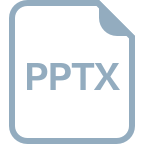
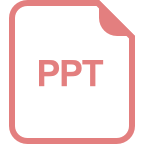













