<?xml version="1.0" encoding="utf-8" ?> <!--<!DOCTYPE web-app PUBLIC--> <!-- "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"--> <!-- "http://java.sun.com/dtd/web-app_2_3.dtd" >--> <web-app> <display-name>Archetype Created Web Application</display-name> <!--1.1 Web前端控制器——》SpringMVC.xml--> <servlet> <servlet-name>dispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>classpath:Work3.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>dispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
时间: 2023-10-29 15:06:55 浏览: 52
这是一个 Java 的 web.xml 配置文件,用于配置一个 web 应用程序。其中包含了 Servlet 配置和映射信息,也包含了 Spring MVC 的配置信息。具体来说:
- `<servlet>` 标签定义了一个 Servlet,其名称为 `dispatcherServlet`,使用的类是 `org.springframework.web.servlet.DispatcherServlet`。初始化参数 `contextConfigLocation` 指定了 Spring MVC 的配置文件为 `classpath:Work3.xml`。
- `<servlet-mapping>` 标签定义了将 `/` URL 映射到 `dispatcherServlet` Servlet 上,也就是说所有的请求都将通过 `dispatcherServlet` 进行处理。
该配置文件的作用是将所有的请求交由 Spring MVC 的 DispatcherServlet 进行处理,并且使用的 Spring MVC 配置文件为 `Work3.xml`。
相关问题
web.xml无法声明元素 web-app
如果您无法在 web.xml 文件中声明元素 web-app,那么可能是以下原因之一:
1. 缺少 XML 头信息:web.xml 文件必须包含 XML 头信息,指定 XML 版本和字符编码。例如:
```
<?xml version="1.0" encoding="UTF-8"?>
```
2. 未指定正确的 DTD:web.xml 文件必须指定正确的 DTD(Document Type Definition),以便验证 XML 的有效性。例如:
```
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd">
```
3. 文件名错误:请确保您的 web.xml 文件名正确,并且位于 WEB-INF 目录下。
如果您已经检查了上述问题,仍然无法声明元素 web-app,请提供更具体的错误信息或上下文,以便更好地帮助您解决问题。
web.xml ejb-jar.xml 示例
下面是web.xml文件和ejb-jar.xml文件的基本结构示例:
web.xml文件示例:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<servlet>
<servlet-name>HelloServlet</servlet-name>
<servlet-class>com.example.HelloServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>HelloServlet</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
<listener>
<listener-class>com.example.MyServletContextListener</listener-class>
</listener>
<error-page>
<error-code>404</error-code>
<location>/error404.jsp</location>
</error-page>
</web-app>
```
ejb-jar.xml文件示例:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE ejb-jar PUBLIC "-//Sun Microsystems, Inc.//DTD Enterprise JavaBeans 2.0//EN"
"http://java.sun.com/dtd/ejb-jar_2_0.dtd">
<ejb-jar>
<enterprise-beans>
<session>
<ejb-name>HelloEJB</ejb-name>
<home>com.example.HelloEJBHome</home>
<remote>com.example.HelloEJB</remote>
<ejb-class>com.example.HelloEJBBean</ejb-class>
</session>
</enterprise-beans>
</ejb-jar>
```
在这两个示例中,web.xml文件定义了一个Servlet、Servlet映射、ServletContextListener和错误页面,而ejb-jar.xml文件定义了一个Session Bean。在实际开发中,这些文件中的具体内容会根据项目需要进行更改和扩展。
相关推荐
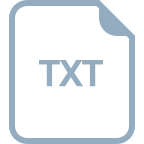
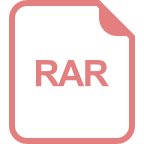
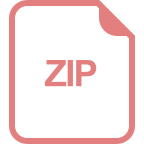












