用Python实现根据公式算出半径为1.0的球面上的点,并写入obj文件
时间: 2024-02-01 10:15:37 浏览: 16
首先,我们需要安装一个名为 `numpy` 的 Python 库来进行数学计算,并且需要手动创建一个 `.obj` 文件来存储点的坐标。
安装 `numpy` 库的命令如下:
```python
pip install numpy
```
接下来是代码实现:
```python
import numpy as np
# 计算球面上的点
def create_sphere_points(radius, latitude, longitude):
phi = np.linspace(0, np.pi, latitude)
theta = np.linspace(0, 2 * np.pi, longitude)
phi, theta = np.meshgrid(phi, theta)
x = radius * np.sin(phi) * np.cos(theta)
y = radius * np.sin(phi) * np.sin(theta)
z = radius * np.cos(phi)
return np.stack((x.flatten(), y.flatten(), z.flatten()), axis=-1)
# 写入到obj文件中
def write_to_file(points, filename):
with open(filename, 'w') as f:
for p in points:
f.write("v {} {} {}\n".format(p[0], p[1], p[2]))
# 设置球体参数
radius = 1.0
latitude = 20
longitude = 20
# 创建球面上的点
points = create_sphere_points(radius, latitude, longitude)
# 写入到obj文件中
write_to_file(points, "sphere.obj")
```
运行代码后,会在当前目录下生成一个名为 `sphere.obj` 的文件,里面保存着球面上的点的坐标。可以使用3D建模软件打开该文件来查看生成的球体。
相关推荐
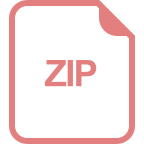













