c语言调用dll与c++调用dll
时间: 2023-09-05 09:09:55 浏览: 219
C语言和C++语言都可以调用DLL(动态链接库),但是两者调用DLL的方式有所不同。
C语言调用DLL需要定义函数指针,并通过LoadLibrary和GetProcAddress函数来获取DLL中的函数地址,再通过函数指针来调用DLL中的函数。例如:
```c
#include <windows.h>
#include <stdio.h>
typedef int (*MYFUNC)(int);
int main()
{
HINSTANCE hInst = LoadLibrary("mydll.dll");
if (hInst == NULL) {
printf("LoadLibrary failed.\n");
return 1;
}
MYFUNC myFunc = (MYFUNC)GetProcAddress(hInst, "myfunc");
if (myFunc == NULL) {
printf("GetProcAddress failed.\n");
return 1;
}
int result = myFunc(10);
printf("result: %d\n", result);
FreeLibrary(hInst);
return 0;
}
```
C++语言调用DLL也需要定义函数指针,但是可以使用extern "C"来指定函数为C语言风格的函数,这样可以避免函数名被C++编译器进行名称修饰。例如:
```c++
#include <windows.h>
#include <iostream>
extern "C" {
typedef int (*MYFUNC)(int);
}
int main()
{
HINSTANCE hInst = LoadLibrary("mydll.dll");
if (hInst == NULL) {
std::cout << "LoadLibrary failed." << std::endl;
return 1;
}
MYFUNC myFunc = (MYFUNC)GetProcAddress(hInst, "myfunc");
if (myFunc == NULL) {
std::cout << "GetProcAddress failed." << std::endl;
return 1;
}
int result = myFunc(10);
std::cout << "result: " << result << std::endl;
FreeLibrary(hInst);
return 0;
}
```
阅读全文
相关推荐
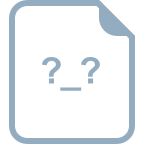
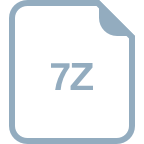
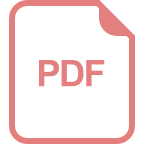















