mfc调用c语言dll,C/C++中动态链接库的创建和调用
时间: 2023-07-16 14:15:17 浏览: 269
动态链接库是一种可重定位的二进制文件,它包含了一些可供其他程序调用的函数或数据。在 Windows 平台上,动态链接库采用 .dll 后缀名,而在 Linux 平台上则采用 .so 后缀名。在本文中,我们将介绍如何使用 MFC 调用 C 语言编写的动态链接库。
# 创建动态链接库
在 Windows 平台上,可以使用 Visual Studio 创建动态链接库。下面是一个简单的示例:
```c
// mydll.h
#ifndef MYDLL_H
#define MYDLL_H
#ifdef __cplusplus
extern "C" {
#endif
__declspec(dllexport) int add(int a, int b);
#ifdef __cplusplus
}
#endif
#endif // MYDLL_H
// mydll.c
#include "mydll.h"
int add(int a, int b)
{
return a + b;
}
```
这个动态链接库包含一个 add 函数,可以对两个整数求和。
# 调用动态链接库
在 MFC 项目中调用动态链接库,需要进行以下几个步骤:
1. 定义一个函数指针类型,指向动态链接库中的函数。
```c++
typedef int (*AddFunc)(int, int);
```
2. 加载动态链接库。
```c++
HINSTANCE hinstLib = LoadLibrary(TEXT("mydll.dll"));
if (hinstLib == NULL) {
AfxMessageBox(TEXT("Failed to load library."));
return;
}
```
3. 获取动态链接库中的函数地址。
```c++
AddFunc addFunc = (AddFunc)GetProcAddress(hinstLib, "add");
if (addFunc == NULL) {
AfxMessageBox(TEXT("Failed to get function address."));
FreeLibrary(hinstLib);
return;
}
```
4. 调用动态链接库中的函数。
```c++
int result = addFunc(1, 2);
CString str;
str.Format(TEXT("1 + 2 = %d"), result);
AfxMessageBox(str);
```
5. 卸载动态链接库。
```c++
FreeLibrary(hinstLib);
```
完整的 MFC 代码示例:
```c++
typedef int (*AddFunc)(int, int);
void CMyDlg::OnButton1()
{
HINSTANCE hinstLib = LoadLibrary(TEXT("mydll.dll"));
if (hinstLib == NULL) {
AfxMessageBox(TEXT("Failed to load library."));
return;
}
AddFunc addFunc = (AddFunc)GetProcAddress(hinstLib, "add");
if (addFunc == NULL) {
AfxMessageBox(TEXT("Failed to get function address."));
FreeLibrary(hinstLib);
return;
}
int result = addFunc(1, 2);
CString str;
str.Format(TEXT("1 + 2 = %d"), result);
AfxMessageBox(str);
FreeLibrary(hinstLib);
}
```
阅读全文
相关推荐
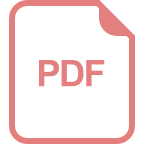
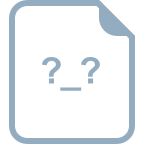
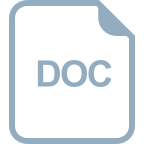















