Java选择课程代码
时间: 2023-07-09 16:11:58 浏览: 186
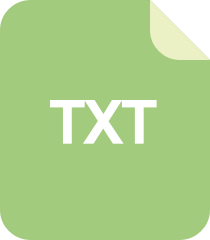
Java学生选课代码
以下是一个简单的 Java 选课系统的代码示例,仅供参考:
Course.java
```
public class Course {
private String id; // 课程编号
private String name; // 课程名称
private int selectedCount; // 已选人数
private int maxCount; // 最大可选人数
private String teacher; // 授课教师
private boolean isSelected; // 是否已选
public Course(String id, String name, int maxCount, String teacher) {
this.id = id;
this.name = name;
this.maxCount = maxCount;
this.teacher = teacher;
this.isSelected = false;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getSelectedCount() {
return selectedCount;
}
public int getMaxCount() {
return maxCount;
}
public String getTeacher() {
return teacher;
}
public boolean isSelected() {
return isSelected;
}
public void setSelected(boolean selected) {
isSelected = selected;
}
public boolean isFull() {
return selectedCount >= maxCount;
}
public boolean select() {
if (isFull()) {
return false;
}
selectedCount++;
isSelected = true;
return true;
}
public boolean cancelSelect() {
if (!isSelected) {
return false;
}
selectedCount--;
isSelected = false;
return true;
}
@Override
public String toString() {
return id + " " + name + " " + selectedCount + "/" + maxCount + " " + teacher;
}
}
```
Student.java
```
public class Student {
private String id; // 学号
private String name; // 姓名
private String password; // 密码
private String grade; // 年级
public Student(String id, String name, String password, String grade) {
this.id = id;
this.name = name;
this.password = password;
this.grade = grade;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getPassword() {
return password;
}
public String getGrade() {
return grade;
}
@Override
public String toString() {
return id + " " + name + " " + grade;
}
}
```
CourseDB.java
```
import java.util.ArrayList;
import java.util.List;
public class CourseDB {
private static CourseDB instance;
private List<Course> courses;
private CourseDB() {
courses = new ArrayList<>();
courses.add(new Course("C001", "Java基础", 30, "张三"));
courses.add(new Course("C002", "Java高级", 20, "李四"));
courses.add(new Course("C003", "数据库", 25, "王五"));
courses.add(new Course("C004", "计算机网络", 20, "赵六"));
courses.add(new Course("C005", "操作系统", 30, "钱七"));
}
public static CourseDB getInstance() {
if (instance == null) {
instance = new CourseDB();
}
return instance;
}
public List<Course> getCourses() {
return courses;
}
public Course findCourseById(String courseId) {
for (Course course : courses) {
if (course.getId().equals(courseId)) {
return course;
}
}
return null;
}
}
```
StudentDB.java
```
import java.util.ArrayList;
import java.util.List;
public class StudentDB {
private static StudentDB instance;
private List<Student> students;
private StudentDB() {
students = new ArrayList<>();
students.add(new Student("S001", "张三", "123456", "大一"));
students.add(new Student("S002", "李四", "123456", "大二"));
students.add(new Student("S003", "王五", "123456", "大三"));
students.add(new Student("S004", "赵六", "123456", "大四"));
students.add(new Student("S005", "钱七", "123456", "大一"));
}
public static StudentDB getInstance() {
if (instance == null) {
instance = new StudentDB();
}
return instance;
}
public List<Student> getStudents() {
return students;
}
public Student findStudentById(String studentId) {
for (Student student : students) {
if (student.getId().equals(studentId)) {
return student;
}
}
return null;
}
}
```
Login.java
```
import java.util.Scanner;
public class Login {
public static Student doLogin() {
Scanner scanner = new Scanner(System.in);
System.out.println("学生选课管理——登录");
System.out.print("请输入学号:");
String studentId = scanner.nextLine();
System.out.print("请输入密码:");
String password = scanner.nextLine();
Student student = StudentDB.getInstance().findStudentById(studentId);
if (student == null || !student.getPassword().equals(password)) {
System.out.println("登录失败,请检查学号和密码是否正确!");
return null;
}
System.out.println("登录成功,欢迎" + student.getName() + "!");
return student;
}
}
```
SelectCourse.java
```
import java.util.Scanner;
public class SelectCourse {
public static void doSelect(Student student) {
Scanner scanner = new Scanner(System.in);
CourseDB courseDB = CourseDB.getInstance();
System.out.println("可选课程列表:");
for (Course course : courseDB.getCourses()) {
System.out.println(course);
}
System.out.print("请输入要选择的课程编号:");
String courseId = scanner.nextLine();
Course course = courseDB.findCourseById(courseId);
if (course == null) {
System.out.println("选课失败,该课程不存在!");
return;
}
if (course.isSelected()) {
System.out.println("选课失败,该课程已经被选择!");
return;
}
boolean success = course.select();
if (success) {
System.out.println("选课成功!");
student.getCourses().add(course);
} else {
System.out.println("选课失败,该课程已经选满!");
}
}
public static void doCancelSelect(Student student) {
Scanner scanner = new Scanner(System.in);
System.out.println("已选课程列表:");
for (Course course : student.getCourses()) {
System.out.println(course);
}
System.out.print("请输入要取消选择的课程编号:");
String courseId = scanner.nextLine();
Course course = CourseDB.getInstance().findCourseById(courseId);
if (course == null) {
System.out.println("取消选课失败,该课程不存在!");
return;
}
boolean success = course.cancelSelect();
if (success) {
System.out.println("取消选课成功!");
student.getCourses().remove(course);
} else {
System.out.println("取消选课失败,该课程未被选择!");
}
}
}
```
MainProcess.java
```
import java.util.Scanner;
public class MainProcess {
public static void main(String[] args) {
Student student = Login.doLogin();
if (student == null) {
return;
}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择要进行的操作:");
System.out.println("1. 选课");
System.out.println("2. 取消选课");
System.out.println("3. 查看已选课程");
System.out.println("4. 退出");
System.out.print("请输入操作编号:");
int choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
SelectCourse.doSelect(student);
break;
case 2:
SelectCourse.doCancelSelect(student);
break;
case 3:
System.out.println("已选课程列表:");
for (Course course : student.getCourses()) {
System.out.println(course);
}
break;
case 4:
System.out.println("退出成功,欢迎下次再来!");
return;
default:
System.out.println("无效的操作编号,请重新输入!");
}
}
}
}
```
以上代码仅供参考,实际运行效果可能会有所不同,您可以根据自己的实际需求和情况进行修改和完善。
阅读全文
相关推荐
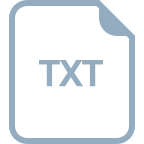
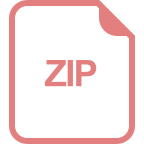
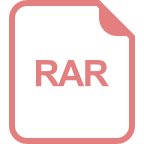
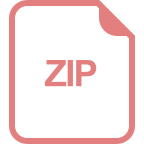
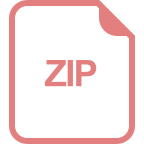
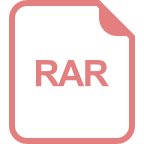
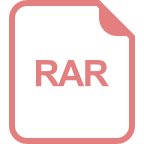
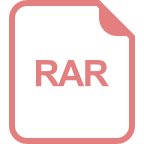
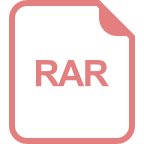
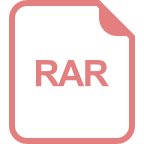
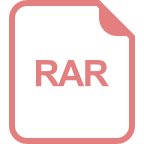
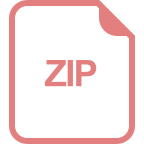
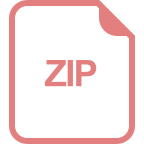
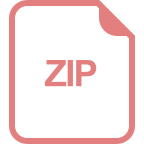