QCustomPlot绘制图表,实现如下功能,鼠标左键框选放大,鼠标右键点击菜单,鼠标右键按下可拖动图标上下平移,使用C++语言,添加完整中文注释
时间: 2024-03-27 19:38:57 浏览: 68
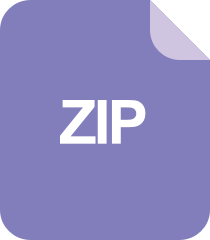
QCustomPlot绘制
好的,您的问题是如何在使用QCustomPlot库绘制图表时实现鼠标左键框选放大和鼠标右键拖动图表上下平移的功能,同时添加完整中文注释。
首先,QCustomPlot是一个强大的Qt绘图库,支持多种类型的图表绘制,例如线图、柱状图、散点图等等。在使用QCustomPlot绘制图表时,我们需要先创建一个QCustomPlot对象,并将其加入到QWidget中,然后通过调用QCustomPlot对象的函数来设置图表的各种属性,例如坐标轴范围、线条颜色等等。
接下来,我们来看如何实现鼠标左键框选放大和鼠标右键拖动图表上下平移的功能。这里我们需要重载QCustomPlot对象的鼠标事件处理函数,以捕捉鼠标事件,然后根据不同的事件类型进行相应的处理。
具体实现方式如下:
```cpp
#include <QCustomPlot>
class MyCustomPlot : public QCustomPlot
{
public:
MyCustomPlot(QWidget *parent = nullptr) : QCustomPlot(parent)
{
// 设置默认的鼠标交互模式为拖拽
setInteractions(QCP::iRangeDrag | QCP::iRangeZoom);
// 设置鼠标滚轮缩放
setInteraction(QCP::iRangeZoom, true);
// 设置鼠标右键菜单
setContextMenuPolicy(Qt::CustomContextMenu);
connect(this, SIGNAL(customContextMenuRequested(QPoint)), this, SLOT(contextMenuRequest(QPoint)));
}
protected:
void mousePressEvent(QMouseEvent *event) override
{
if (event->button() == Qt::LeftButton) {
// 鼠标左键按下,记录起始点
m_startPos = event->pos();
}
else if (event->button() == Qt::RightButton) {
// 鼠标右键按下,记录起始位置
m_lastPos = event->pos();
}
QCustomPlot::mousePressEvent(event);
}
void mouseReleaseEvent(QMouseEvent *event) override
{
if (event->button() == Qt::LeftButton && event->pos() != m_startPos) {
// 鼠标左键释放,计算选框的范围,并放大选框中的部分
QRect zoomRect = QRect(m_startPos, event->pos()).normalized();
zoomIn(zoomRect);
}
else if (event->button() == Qt::RightButton) {
// 鼠标右键释放,计算拖动的距离并平移图表
QPoint delta = event->pos() - m_lastPos;
scroll(delta.x(), delta.y());
}
QCustomPlot::mouseReleaseEvent(event);
}
private slots:
void contextMenuRequest(QPoint pos)
{
QMenu *menu = new QMenu(this);
menu->addAction(tr("重置缩放"), this, SLOT(resetZoom()));
menu->popup(mapToGlobal(pos));
}
private:
QPoint m_startPos; // 记录鼠标左键按下的起始位置
QPoint m_lastPos; // 记录鼠标右键按下的起始位置
};
```
在上面的代码中,我们创建了一个名为MyCustomPlot的类,继承自QCustomPlot,并重载了鼠标事件处理函数。在鼠标按下事件中,我们记录了鼠标左键和右键按下的起始位置;在鼠标释放事件中,如果是鼠标左键释放,我们计算选框的范围并调用zoomIn函数对选框中的部分进行放大;如果是鼠标右键释放,我们计算拖动的距离并调用scroll函数来平移图表;同时,在右键菜单中添加了一个“重置缩放”的选项,用于将图表恢复到初始状态。
最后,为了方便使用和维护,我们可以在代码中添加完整中文注释,以提高代码的可读性和易用性。
希望这样能够解决您的问题,如有不足之处,还请指出。
阅读全文
相关推荐

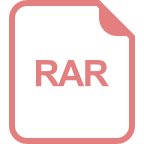
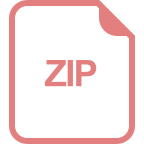
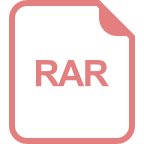
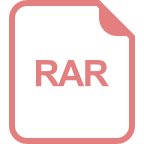
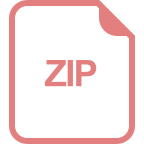
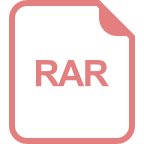
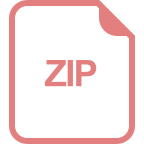
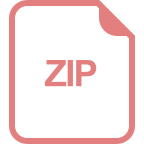
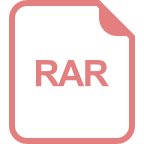
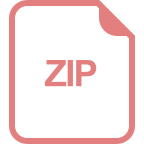
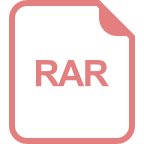
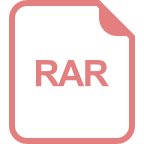
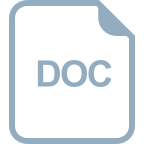
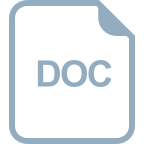