jdbc执行多条sql查询语句
时间: 2023-09-10 09:04:45 浏览: 63
### 回答1:
JDBC允许在一个Statement中执行多条SQL查询语句,这种方式叫做批量操作(batch processing)。使用批量操作,可以减少与数据库的通讯次数,提高效率。
以下是一个批量操作的示例代码:
```
import java.sql.*;
public class BatchExample {
public static void main(String[] args) {
try {
// 创建连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "root", "password");
// 创建Statement
Statement stmt = conn.createStatement();
// 执行多条查询语句
stmt.addBatch("SELECT * FROM mytable WHERE id = 1");
stmt.addBatch("SELECT * FROM mytable WHERE id = 2");
stmt.addBatch("SELECT * FROM mytable WHERE id = 3");
// 执行批量操作
int[] results = stmt.executeBatch();
// 处理查询结果
for (int i = 0; i < results.length; i++) {
ResultSet rs = stmt.getResultSet();
while (rs.next()) {
System.out.println(rs.getInt("id") + ", " + rs.getString("name"));
}
stmt.getMoreResults();
}
// 关闭连接
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,使用Statement的addBatch()方法将多条查询语句添加到批量操作中,然后使用executeBatch()方法执行批量操作,返回一个int类型的数组,数组中的每个元素表示对应查询语句的影响行数或结果集。最后通过getResultSet()方法获取查询结果集,再通过getMoreResults()方法获取下一条查询结果。
### 回答2:
JDBC(Java Database Connectivity)是一种用于执行数据库操作的Java API。在JDBC中,可以使用Statement或者PreparedStatement对象执行SQL查询语句。
要执行多条SQL查询语句,可以通过以下步骤:
1. 创建一个Connection对象,用于与数据库建立连接。
2. 使用Connection对象创建一个Statement对象。
3. 使用Statement对象执行第一条查询语句,并将结果保存在ResultSet对象中。
4. 使用ResultSet对象读取第一条查询结果。
5. 使用Statement对象继续执行第二条查询语句,并将结果保存在新的ResultSet对象中。
6. 使用新的ResultSet对象读取第二条查询结果。
7. 重复步骤5和6,直到执行完所有的查询语句。
8. 关闭ResultSet对象,Statement对象和Connection对象,释放资源。
在代码实现上,可以使用循环来执行多条查询语句,如下所示:
```java
Connection connection = null;
Statement statement = null;
ResultSet resultSet = null;
try {
// 建立数据库连接
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/your_database", "username", "password");
// 创建Statement对象
statement = connection.createStatement();
// 定义多条查询语句
String[] queries = {"SELECT * FROM table1", "SELECT * FROM table2", "SELECT * FROM table3"};
// 循环执行多条查询语句
for (String query : queries) {
// 执行查询语句
resultSet = statement.executeQuery(query);
// 处理查询结果
while (resultSet.next()) {
// 读取查询结果
// ...
}
// 关闭ResultSet对象
resultSet.close();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭Statement和Connection对象
if (statement != null) {
statement.close();
}
if (connection != null) {
connection.close();
}
}
```
上述代码通过循环执行多条查询语句,每次执行完一条查询语句后关闭ResultSet对象,最终在finally块中关闭Statement和Connection对象,确保资源的正确释放。
### 回答3:
JDBC(Java Database Connectivity)是一种用于在Java程序中与数据库进行连接和交互的API(Application Programming Interface)。JDBC允许我们通过执行SQL语句来操作数据库。
如果要执行多条SQL查询语句,可以通过使用Java中的JDBC批处理来实现。批处理允许我们一次性发送多个SQL语句到数据库执行,并且可以减少与数据库的往返次数,提高性能。
要执行多条SQL查询语句,可以按照以下步骤:
1. 获取数据库连接:使用JDBC连接数据库,并获取一个数据库连接对象。
2. 创建Statement对象:使用连接对象创建一个Statement对象,用于执行SQL语句。
3. 添加SQL查询语句:使用Statement对象的addBatch方法,将多条SQL查询语句添加到批处理中。
4. 执行批处理:使用Statement对象的executeBatch方法,执行SQL查询语句的批处理。
5. 处理结果:使用Statement对象的getResultSet方法,获取SQL查询语句的执行结果集,并进行后续的处理。
示例代码如下:
```java
// 步骤1:获取数据库连接
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
// 步骤2:创建Statement对象
Statement statement = connection.createStatement();
// 步骤3:添加SQL查询语句
statement.addBatch("SELECT * FROM table1");
statement.addBatch("SELECT * FROM table2");
statement.addBatch("SELECT * FROM table3");
// 步骤4:执行批处理
int[] results = statement.executeBatch();
// 步骤5:处理结果
for (int result : results) {
if (result == Statement.SUCCESS_NO_INFO || result > 0) {
ResultSet resultSet = statement.getResultSet();
// 处理resultSet
} else {
// 出错处理
}
}
// 关闭连接和资源
statement.close();
connection.close();
```
通过以上步骤,我们可以使用JDBC执行多条SQL查询语句,并处理查询结果。使用JDBC批处理可以提高执行效率,并减少与数据库的通信次数。
相关推荐
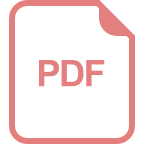
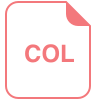
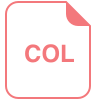
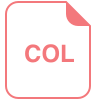
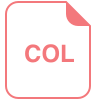
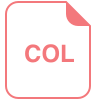









