用Python写一个赛车游戏
时间: 2024-03-30 21:02:58 浏览: 83
好的,以下是一个简单的赛车游戏的Python代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 创建屏幕
screen = pygame.display.set_mode((800, 600))
# 游戏标题和图标
pygame.display.set_caption("Race Car")
icon = pygame.image.load("car.png")
pygame.display.set_icon(icon)
# 赛车图片
car_image = pygame.image.load("racecar.png")
car_width = 70
# 障碍物
obstacle_image = pygame.image.load("obstacle.png")
obstacle_width = 70
obstacle_height = 70
# 颜色
white = (255, 255, 255)
black = (0, 0, 0)
# 字体
font = pygame.font.SysFont(None, 25)
# 显示分数
def display_score(score):
score_text = font.render("Score: " + str(score), True, black)
screen.blit(score_text, (0, 0))
# 显示障碍物
def display_obstacle(obstacle_x, obstacle_y):
screen.blit(obstacle_image, (obstacle_x, obstacle_y))
# 碰撞检测
def is_collision(car_x, car_y, obstacle_x, obstacle_y):
distance = ((car_x - obstacle_x) ** 2 + (car_y - obstacle_y) ** 2) ** 0.5
if distance < (car_width + obstacle_width) / 2:
return True
else:
return False
# 游戏循环
def game_loop():
# 赛车初始位置
car_x = 400 - car_width / 2
car_y = 500
# 障碍物初始位置
obstacle_x = random.randint(0, 800 - obstacle_width)
obstacle_y = -obstacle_height
# 障碍物速度
obstacle_speed = 5
# 分数
score = 0
# 游戏结束标志
game_over = False
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# 移动赛车
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
car_x -= 50
elif event.key == pygame.K_RIGHT:
car_x += 50
# 背景
screen.fill(white)
# 显示赛车
screen.blit(car_image, (car_x, car_y))
# 显示障碍物
display_obstacle(obstacle_x, obstacle_y)
# 显示分数
display_score(score)
# 障碍物移动
obstacle_y += obstacle_speed
# 障碍物超出屏幕
if obstacle_y > 600:
obstacle_x = random.randint(0, 800 - obstacle_width)
obstacle_y = -obstacle_height
score += 1
obstacle_speed += 1
# 碰撞检测
if is_collision(car_x, car_y, obstacle_x, obstacle_y):
game_over = True
# 更新屏幕
pygame.display.update()
# 退出Pygame
pygame.quit()
quit()
# 开始游戏循环
game_loop()
```
这个游戏中,玩家控制一辆赛车,避开障碍物并尽可能地获得高分。游戏循环中包含了移动赛车、移动障碍物、检测碰撞等逻辑,同时还有分数统计和游戏结束标志。游戏的画面使用Pygame来绘制,包括赛车、障碍物、背景和分数。
相关推荐
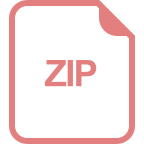
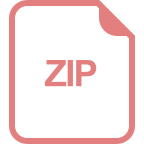














