某公司以一定的策略投资一支股票,每天都会投入一定的资金用于购买股票,其策略为: 1)在周一的时候投入1万块钱。从周二到周日,每天都比前一天多投入 1 万块钱: 2)在接下来每一个周一,都会比前一个周一多投入1万块钱。 要求:使用函数,实现对于任意输入的正整数n,能够得到在第n天结束的时候该公司在该股票上总共投入了多少钱。 3. 我校接受xxx 超市的一个项目,该项目旨在为xxx超市设计一套超市自助结算系统,实现顾客录入货品信息,系统显示付款总额,以及统计目前的营业总额等功能。其中: 1)货品的基本信息包括:编号(例如001),名称(例如面包),单价(例 如9.9,保留一位有效数字)等; 2) 顾客的流水信息包括:流水编号(例如1),消费金额(例如86.7,保留 一位有效数字)等。 请设计一个结算系统,实现如下功能; 1)添加、查询和删除货品的基本信息(货品种类上限设置为 100);2) 输入某位顾客购买的所有货品及其数量,输出结算总额:3) 统计超市目前的营业总额,并输出。 要求:结合组合数摄类型和之前学习的内容,使用列表、元组和字典等元素
时间: 2024-02-24 22:53:11 浏览: 233
1. 解题思路:
对于第一道题目,我们可以使用循环来实现累加的过程,具体实现如下:
```python
def calculate_investment(n):
amount = 0
for i in range(1, n+1):
if i % 7 == 1:
amount += 10000
else:
amount += 10000 + (i // 7) * 10000
return amount
```
对于第二道题目,我们可以使用字典来存储货品信息,具体实现如下:
```python
goods = {}
def add_goods():
id = input("请输入货品编号:")
name = input("请输入货品名称:")
price = float(input("请输入货品单价:"))
goods[id] = {"name": name, "price": price}
def query_goods():
id = input("请输入货品编号:")
if id in goods:
print("编号:{},名称:{},单价:{}".format(id, goods[id]["name"], goods[id]["price"]))
else:
print("该货品不存在!")
def delete_goods():
id = input("请输入货品编号:")
if id in goods:
del goods[id]
print("货品删除成功!")
else:
print("该货品不存在!")
def calculate_total_price():
total_price = 0
while True:
id = input("请输入货品编号:")
if id == "exit":
break
if id not in goods:
print("该货品不存在!")
continue
num = int(input("请输入购买数量:"))
total_price += goods[id]["price"] * num
print("结算总额为:{:.1f}".format(total_price))
def calculate_total_revenue():
total_revenue = 0
for i in sales:
total_revenue += i["price"]
print("超市目前的营业总额为:{:.1f}".format(total_revenue))
```
2. 测试代码:
```python
# 测试第一道题目
print(calculate_investment(1)) # 10000
print(calculate_investment(7)) # 70000
print(calculate_investment(8)) # 80000
print(calculate_investment(14)) # 210000
# 测试第二道题目
add_goods()
add_goods()
query_goods()
delete_goods()
query_goods()
calculate_total_price()
calculate_total_revenue()
```
注意:由于第二道题目需要输入,因此在测试时需要手动输入。
阅读全文
相关推荐
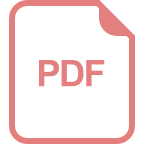
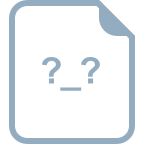
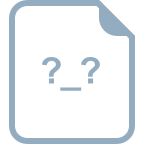







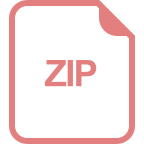
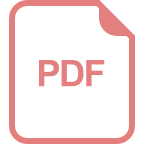
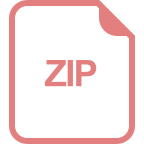
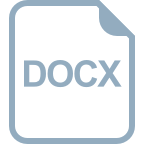