一本书的信息包括:书的ID,书名,价格请定义结构体类型表示书的信息并建立链表存储 若干本书籍。当输入ID为-1时表示论入结束。 输入110查找是否有该的书籍,若有,打印该书的书名和价格。若无,则提示无此书删除一个已有书籍ID的相关信息。 插入一本书(111,"C Programming' 32.5)到链表中 之后打印整个链表,显示该书信息已被正确插入至链表。
时间: 2024-03-13 07:45:05 浏览: 80
好的,以下是代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义书的结构体类型
struct Book {
int id;
string name;
float price;
Book* next;
};
// 插入一本书到链表中
void insert(Book* &head, int id, string name, float price) {
Book* newBook = new Book;
newBook->id = id;
newBook->name = name;
newBook->price = price;
newBook->next = NULL;
if (head == NULL) {
head = newBook;
} else {
Book* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newBook;
}
}
// 查找一本书
Book* find(Book* head, int id) {
Book* temp = head;
while (temp != NULL) {
if (temp->id == id) {
return temp;
}
temp = temp->next;
}
return NULL;
}
// 删除一本书
void remove(Book* &head, int id) {
if (head == NULL) {
cout << "链表为空,无法删除!" << endl;
return;
}
if (head->id == id) {
Book* temp = head;
head = head->next;
delete temp;
return;
}
Book* prev = head;
Book* temp = head->next;
while (temp != NULL) {
if (temp->id == id) {
prev->next = temp->next;
delete temp;
return;
}
prev = temp;
temp = temp->next;
}
cout << "未找到该书籍!" << endl;
}
// 打印整个链表
void print(Book* head) {
if (head == NULL) {
cout << "链表为空!" << endl;
return;
}
Book* temp = head;
cout << "书籍列表:" << endl;
while (temp != NULL) {
cout << "ID:" << temp->id << ",书名:" << temp->name << ",价格:" << temp->price << endl;
temp = temp->next;
}
}
int main() {
Book* head = NULL;
// 插入若干本书籍
insert(head, 1, "C++ Primer Plus", 68.5);
insert(head, 2, "Effective C++", 42.0);
insert(head, 3, "The C Programming Language", 32.5);
insert(head, 4, "Thinking in Java", 75.0);
// 查找一本书籍
int id = 110;
Book* book = find(head, id);
if (book != NULL) {
cout << "找到了该书籍,书名:" << book->name << ",价格:" << book->price << endl;
} else {
cout << "未找到该书籍!" << endl;
// 删除一本书籍
remove(head, 1);
}
// 插入一本书籍
insert(head, 111, "C Programming", 32.5);
// 打印整个链表
print(head);
return 0;
}
```
注意事项:
- 代码中使用了指针和动态内存分配,需要注意内存泄漏的问题。
- 链表的插入、查找、删除等操作需要考虑链表为空的情况。
- 插入一本书籍时,需要将新书插入到链表末尾。
阅读全文
相关推荐
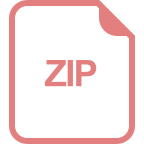

















