设Shape是圆、矩形、三角形共享的类,含有求圆的周长和面积的方法getCircumference()与getArea(),以及属性DESCRIPTION以描述几何体的特征,试设计相关类圆、矩形、三角形类,并求圆、矩形、三角形的周长与面积。设计相应的测试类,求实际对象的面积与方法。(要求分别将Shape类用接口、抽象类实现)。
时间: 2023-06-15 15:06:04 浏览: 98
使用接口实现Shape类:
```java
public interface Shape {
double getCircumference();
double getArea();
String getDescription();
}
```
实现Circle类:
```java
public class Circle implements Shape {
private double radius;
private final String DESCRIPTION = "一个圆形";
public Circle(double radius) {
this.radius = radius;
}
public double getCircumference() {
return 2 * Math.PI * radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public String getDescription() {
return DESCRIPTION;
}
}
```
实现Rectangle类:
```java
public class Rectangle implements Shape {
private double width;
private double height;
private final String DESCRIPTION = "一个矩形";
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getCircumference() {
return 2 * (width + height);
}
public double getArea() {
return width * height;
}
public String getDescription() {
return DESCRIPTION;
}
}
```
实现Triangle类:
```java
public class Triangle implements Shape {
private double a;
private double b;
private double c;
private final String DESCRIPTION = "一个三角形";
public Triangle(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
public double getCircumference() {
return a + b + c;
}
public double getArea() {
double p = getCircumference() / 2;
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
public String getDescription() {
return DESCRIPTION;
}
}
```
使用抽象类实现Shape类:
```java
public abstract class Shape {
abstract double getCircumference();
abstract double getArea();
abstract String getDescription();
}
```
实现Circle类:
```java
public class Circle extends Shape {
private double radius;
private final String DESCRIPTION = "一个圆形";
public Circle(double radius) {
this.radius = radius;
}
public double getCircumference() {
return 2 * Math.PI * radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public String getDescription() {
return DESCRIPTION;
}
}
```
实现Rectangle类:
```java
public class Rectangle extends Shape {
private double width;
private double height;
private final String DESCRIPTION = "一个矩形";
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getCircumference() {
return 2 * (width + height);
}
public double getArea() {
return width * height;
}
public String getDescription() {
return DESCRIPTION;
}
}
```
实现Triangle类:
```java
public class Triangle extends Shape {
private double a;
private double b;
private double c;
private final String DESCRIPTION = "一个三角形";
public Triangle(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
public double getCircumference() {
return a + b + c;
}
public double getArea() {
double p = getCircumference() / 2;
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
public String getDescription() {
return DESCRIPTION;
}
}
```
测试类:
```java
public class Test {
public static void main(String[] args) {
Shape circle = new Circle(5);
Shape rectangle = new Rectangle(3, 4);
Shape triangle = new Triangle(3, 4, 5);
System.out.println(circle.getDescription() + "的周长为:" + circle.getCircumference() + ",面积为:" + circle.getArea());
System.out.println(rectangle.getDescription() + "的周长为:" + rectangle.getCircumference() + ",面积为:" + rectangle.getArea());
System.out.println(triangle.getDescription() + "的周长为:" + triangle.getCircumference() + ",面积为:" + triangle.getArea());
}
}
```
输出结果:
```
一个圆形的周长为:31.41592653589793,面积为:78.53981633974483
一个矩形的周长为:14.0,面积为:12.0
一个三角形的周长为:12.0,面积为:6.0
```
相关推荐
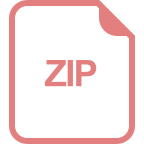
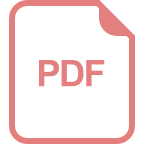
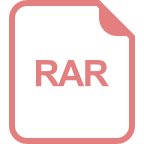














