JAVA用接口、多态、继承、类计算三角形和矩形周长及面积的方法
时间: 2023-12-29 07:05:15 浏览: 83
很高兴回答你的问题!针对你的需求,我给出下面的代码:
// 定义一个接口Shape
interface Shape {
double getArea(); // 计算面积
double getPerimeter(); // 计算周长
}
// 定义一个三角形Triangle类,继承自Shape接口
class Triangle implements Shape {
private double a, b, c; // 三角形三边长
public Triangle(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
// 重写Shape接口的方法
public double getArea() {
double p = (a + b + c) / 2; // 利用海伦公式计算面积
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
public double getPerimeter() {
return a + b + c; // 计算周长
}
}
// 定义一个矩形Rectangle类,继承自Shape接口
class Rectangle implements Shape {
private double width, height; // 矩形长和宽
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
// 重写Shape接口的方法
public double getArea() {
return width * height; // 计算面积
}
public double getPerimeter() {
return (width + height) * 2; // 计算周长
}
}
// 测试代码
public class Test {
public static void main(String[] args) {
Shape[] shapes = new Shape[2];
shapes[0] = new Triangle(3, 4, 5);
shapes[1] = new Rectangle(2, 3);
for (Shape s : shapes) {
System.out.println("面积:" + s.getArea());
System.out.println("周长:" + s.getPerimeter());
}
}
}
希望我的回答能够帮到你!
阅读全文
相关推荐
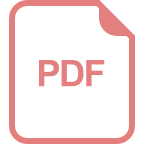
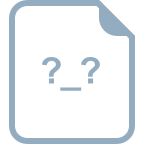


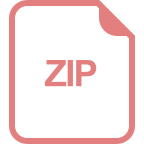
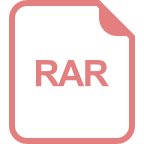
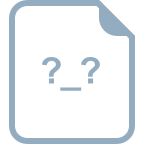
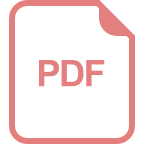
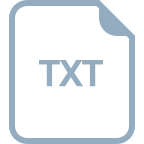
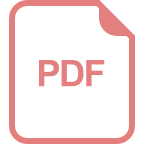
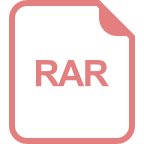






