选择题题库系统
时间: 2023-08-29 22:07:47 浏览: 69
好的,下面是一个简单的选择题题库系统示例,你可以在 Python 中运行它:
```python
class Question:
def __init__(self, text, choices, answer):
self.text = text
self.choices = choices
self.answer = answer
def check_answer(self, answer):
return self.answer == answer
class Quiz:
def __init__(self, questions):
self.questions = questions
self.score = 0
self.question_index = 0
def get_question(self):
return self.questions[self.question_index]
def display_question(self):
question = self.get_question()
print(f"Question {self.question_index + 1}: {question.text}")
for i, choice in enumerate(question.choices):
print(f"{i + 1}: {choice}")
answer = input("Enter your choice (1-4): ")
self.check_answer(answer)
self.question_index += 1
def check_answer(self, answer):
question = self.get_question()
if question.check_answer(int(answer)):
self.score += 1
print("Correct!")
else:
print("Incorrect!")
def display_score(self):
print(f"Your score is {self.score}/{len(self.questions)}")
question_bank = [
Question("What is the capital of France?", ["Paris", "London", "Berlin", "Madrid"], 1),
Question("What is the largest country in the world?", ["Russia", "USA", "China", "India"], 1),
Question("What is the highest mountain in the world?", ["Mount Everest", "Mount Kilimanjaro", "Mount Fuji", "Mount McKinley"], 1),
]
quiz = Quiz(question_bank)
while quiz.question_index < len(quiz.questions):
quiz.display_question()
quiz.display_score()
```
该程序定义了 `Question` 和 `Quiz` 两个类,其中 `Question` 表示每个选择题,包含题目文本、选项和正确答案;`Quiz` 表示整个题库,包含多个题目和用户的得分。在程序中,我们创建了一个包含多个 `Question` 对象的 `question_bank` 列表,并将其传递给 `Quiz` 对象。`Quiz` 对象中的 `display_question()` 方法会显示当前问题的文本和选项,并要求用户输入答案,然后调用 `check_answer()` 方法来检查答案是否正确。在程序的主循环中,我们一直调用 `display_question()` 直到所有问题都回答完毕,最后调用 `display_score()` 方法显示用户的得分。注意,这个程序只是一个简单示例,如果你需要更完整的选择题题库系统,你需要考虑更多的边界条件和错误处理。
相关推荐
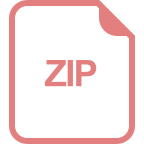
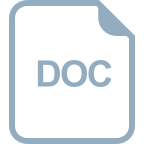














