将代码的gets方法改为getchar方法:#include<iostream> #include<string> #include<bits/stdc++.h> using namespace std; const int N = 1e6; int trie[N + 50][26]; int num[N + 50] = { 0 }; int pos = 1; void Insert(char str[]) { int index = 0; for (int i = 0; str[i]; i++) { int n = str[i] - 'a'; if (trie[index][n] == 0) trie[index][n] = pos++; index = trie[index][n]; num[index]++; } } int Find(char str[]) { int index = 0; for (int i = 0; str[i]; i++) { int n = str[i] - 'a'; if (trie[index][n] == 0) return 0; index = trie[index][n]; } return num[index]; } int main() { char str[11]; while (gets(str)) { if (!strlen(str)) break; Insert(str); } while (gets(str)) { int res = Find(str); if (res > 0) cout << "YES" << endl; else cout << "NO" << endl; } return 0; }
时间: 2024-04-23 08:26:38 浏览: 11
好的,代码的修改如下:
```c++
#include<iostream>
#include<string>
#include<bits/stdc++.h>
using namespace std;
const int N = 1e6;
int trie[N + 50][26];
int num[N + 50] = { 0 };
int pos = 1;
void Insert(char str[]) {
int index = 0;
for (int i = 0; str[i]; i++) {
int n = str[i] - 'a';
if (trie[index][n] == 0)
trie[index][n] = pos++;
index = trie[index][n];
num[index]++;
}
}
int Find(char str[]) {
int index = 0;
for (int i = 0; str[i]; i++) {
int n = str[i] - 'a';
if (trie[index][n] == 0)
return 0;
index = trie[index][n];
}
return num[index];
}
int main() {
char str[11];
int i = 0;
while ((str[i] = getchar()) != '\n') { // 读入一行
i++;
}
str[i] = '\0';
i = 0;
while (str[i] != '\0') { // 处理每个单词
int j = i;
while (str[j] != ' ' && str[j] != '\0') {
j++;
}
char word[11];
int k = 0;
for (int p = i; p < j; p++) {
word[k++] = str[p];
}
word[k] = '\0';
Insert(word);
i = j + 1;
}
while ((str[i] = getchar()) != EOF) { // 读入查询
if (str[i] == '\n') {
int res = Find(str);
if (res > 0)
cout << "YES" << endl;
else
cout << "NO" << endl;
i = 0;
} else {
i++;
}
}
return 0;
}
```
修改思路:首先需要读入一行字符串,然后逐个处理每个单词,插入到 Trie 树中;之后每次读入查询时,读入一行,然后对每个单词查询 Trie 树,并输出结果。在这个过程中,需要注意每个单词之间的分割符号。
相关推荐
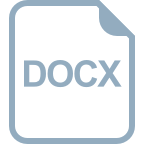
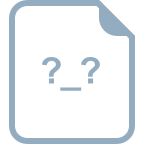
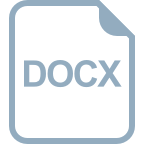















