写一个STM32F103RCT6处理GY-30模块数据的程序
时间: 2023-06-13 22:03:07 浏览: 115
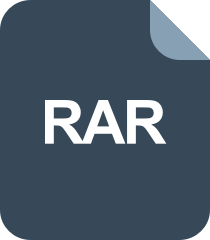
STM32F103RCT6 -time6-20us中断一次,50khz,JSCOPE-RTT组件通过 jlink上传波形
好的,下面是一个简单的程序示例:
```c
#include "stm32f10x.h"
#include "i2c.h"
#include "delay.h"
#include "GY-30.h"
int main(void)
{
uint16_t lux;
// 初始化I2C总线
I2C_Configuration();
// 初始化延时函数
delay_init();
// 初始化GY-30模块
GY30_Init();
while(1)
{
// 读取光照强度值
lux = GY30_ReadLux();
// 延时一段时间
delay_ms(500);
}
}
```
其中,`i2c.h` 和 `delay.h` 是 I2C 和延时函数的头文件,`GY-30.h` 是GY-30模块的头文件。
下面是 `GY-30.h` 的内容:
```c
#ifndef __GY30_H
#define __GY30_H
#include "stm32f10x.h"
#define GY30_ADDR 0x46 // GY-30模块的I2C地址
void GY30_Init(void);
uint16_t GY30_ReadLux(void);
#endif /* __GY30_H */
```
下面是 `GY-30.c` 的内容:
```c
#include "GY-30.h"
static void GY30_WriteReg(uint8_t reg, uint8_t value)
{
I2C_Start();
I2C_SendByte(GY30_ADDR << 1);
I2C_WaitAck();
I2C_SendByte(reg);
I2C_WaitAck();
I2C_SendByte(value);
I2C_WaitAck();
I2C_Stop();
}
static uint8_t GY30_ReadReg(uint8_t reg)
{
uint8_t value;
I2C_Start();
I2C_SendByte(GY30_ADDR << 1);
I2C_WaitAck();
I2C_SendByte(reg);
I2C_WaitAck();
I2C_Start();
I2C_SendByte((GY30_ADDR << 1) | 0x01);
I2C_WaitAck();
value = I2C_ReadByte();
I2C_Stop();
return value;
}
void GY30_Init(void)
{
GY30_WriteReg(0x01, 0x01); // 打开光照强度测量
GY30_WriteReg(0x10, 0x20); // 分辨率设置为1 Lux
}
uint16_t GY30_ReadLux(void)
{
uint16_t lux;
uint8_t val1, val2;
val1 = GY30_ReadReg(0x00); // 读取光照强度值的高八位
val2 = GY30_ReadReg(0x01); // 读取光照强度值的低八位
lux = (val1 << 8) | val2; // 合并高低八位数据
return lux;
}
```
其中,`GY30_WriteReg` 和 `GY30_ReadReg` 函数用于向GY-30模块写入和读取寄存器值,`GY30_Init` 函数用于初始化GY-30模块,`GY30_ReadLux` 函数用于读取光照强度值。
阅读全文
相关推荐
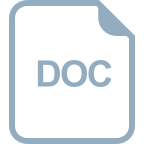
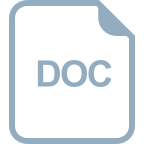
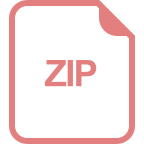
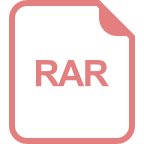
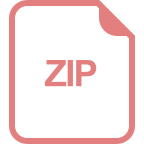
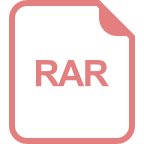
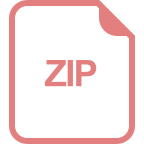
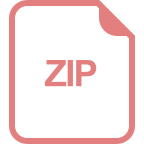






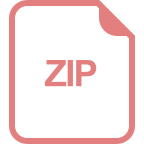
