智能小车基于stm32f103c8t6的电磁循迹代码
时间: 2023-08-31 07:05:23 浏览: 172
电磁循迹是指通过小车底部的电磁感应模块来感知地面上的磁场,从而实现小车沿着磁场走的一种方式。下面是一个基于STM32F103C8T6的电磁循迹代码示例:
```c
#include "stm32f10x.h"
#define LED_PIN GPIO_Pin_13
#define LED_PORT GPIOC
#define LEFT_SENSOR_PIN GPIO_Pin_0
#define LEFT_SENSOR_PORT GPIOA
#define RIGHT_SENSOR_PIN GPIO_Pin_1
#define RIGHT_SENSOR_PORT GPIOA
void delay(int time);
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* 使能GPIOA和GPIOC时钟 */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOC, ENABLE);
/* 配置LED引脚为输出模式 */
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_PORT, &GPIO_InitStructure);
/* 配置左侧电磁感应模块引脚为输入模式 */
GPIO_InitStructure.GPIO_Pin = LEFT_SENSOR_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(LEFT_SENSOR_PORT, &GPIO_InitStructure);
/* 配置右侧电磁感应模块引脚为输入模式 */
GPIO_InitStructure.GPIO_Pin = RIGHT_SENSOR_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(RIGHT_SENSOR_PORT, &GPIO_InitStructure);
while(1)
{
/* 获取左侧电磁感应模块的状态 */
uint8_t left_sensor_status = GPIO_ReadInputDataBit(LEFT_SENSOR_PORT, LEFT_SENSOR_PIN);
/* 获取右侧电磁感应模块的状态 */
uint8_t right_sensor_status = GPIO_ReadInputDataBit(RIGHT_SENSOR_PORT, RIGHT_SENSOR_PIN);
/* 如果左右两侧都没有检测到磁场,则小车前进 */
if(left_sensor_status == 0 && right_sensor_status == 0)
{
GPIO_SetBits(LED_PORT, LED_PIN);
delay(1000); // 延时1s
}
/* 如果左侧检测到磁场,则小车向右转 */
else if(left_sensor_status == 1 && right_sensor_status == 0)
{
GPIO_SetBits(LED_PORT, LED_PIN);
delay(500); // 延时0.5s
}
/* 如果右侧检测到磁场,则小车向左转 */
else if(left_sensor_status == 0 && right_sensor_status == 1)
{
GPIO_SetBits(LED_PORT, LED_PIN);
delay(500); // 延时0.5s
}
/* 如果左右两侧都检测到磁场,则小车停止不动 */
else if(left_sensor_status == 1 && right_sensor_status == 1)
{
GPIO_ResetBits(LED_PORT, LED_PIN);
}
}
}
void delay(int time)
{
int i, j;
for(i = time; i > 0; i--)
{
for(j = 0; j < 1000; j++);
}
}
```
上述代码中,使用了PA0和PA1作为左右两侧电磁感应模块的输入引脚,使用PC13作为LED灯的输出引脚。当左右两侧都没有检测到磁场时,小车会前进;当左侧检测到磁场时,小车会向右转;当右侧检测到磁场时,小车会向左转;当左右两侧都检测到磁场时,小车会停止不动。同时,在代码中还使用了一个简单的延时函数来控制小车转向的时间。
相关推荐
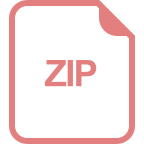
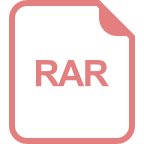
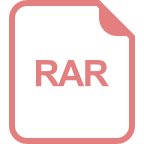














