设计一个图书信息管理系统JAVA 数据库sql
时间: 2023-09-26 22:09:18 浏览: 52
好的,我可以为您提供一个简单的图书信息管理系统的JAVA代码和数据库SQL语句,具体实现如下:
首先,我们需要定义一个Book类,包含书籍的属性和方法:
```java
public class Book {
private int id;
private String title;
private String author;
private String publisher;
private int year;
private int pages;
private double price;
public Book(int id, String title, String author, String publisher, int year, int pages, double price) {
this.id = id;
this.title = title;
this.author = author;
this.publisher = publisher;
this.year = year;
this.pages = pages;
this.price = price;
}
public int getId() {
return id;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getPublisher() {
return publisher;
}
public int getYear() {
return year;
}
public int getPages() {
return pages;
}
public double getPrice() {
return price;
}
}
```
然后,我们需要实现一个BookDAO类,用于管理图书信息的增删改查等操作:
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class BookDAO {
private String jdbcURL;
private String jdbcUsername;
private String jdbcPassword;
private Connection jdbcConnection;
public BookDAO(String jdbcURL, String jdbcUsername, String jdbcPassword) {
this.jdbcURL = jdbcURL;
this.jdbcUsername = jdbcUsername;
this.jdbcPassword = jdbcPassword;
}
private void connect() throws SQLException {
if (jdbcConnection == null || jdbcConnection.isClosed()) {
try {
Class.forName("com.mysql.jdbc.Driver");
} catch (ClassNotFoundException e) {
throw new SQLException(e);
}
jdbcConnection = DriverManager.getConnection(
jdbcURL, jdbcUsername, jdbcPassword);
}
}
private void disconnect() throws SQLException {
if (jdbcConnection != null && !jdbcConnection.isClosed()) {
jdbcConnection.close();
}
}
public boolean insertBook(Book book) throws SQLException {
String sql = "INSERT INTO book (title, author, publisher, year, pages, price) VALUES (?, ?, ?, ?, ?, ?)";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setString(1, book.getTitle());
statement.setString(2, book.getAuthor());
statement.setString(3, book.getPublisher());
statement.setInt(4, book.getYear());
statement.setInt(5, book.getPages());
statement.setDouble(6, book.getPrice());
boolean rowInserted = statement.executeUpdate() > 0;
statement.close();
disconnect();
return rowInserted;
}
public List<Book> listAllBooks() throws SQLException {
List<Book> listBook = new ArrayList<>();
String sql = "SELECT * FROM book";
connect();
Statement statement = jdbcConnection.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
int id = resultSet.getInt("id");
String title = resultSet.getString("title");
String author = resultSet.getString("author");
String publisher = resultSet.getString("publisher");
int year = resultSet.getInt("year");
int pages = resultSet.getInt("pages");
double price = resultSet.getDouble("price");
Book book = new Book(id, title, author, publisher, year, pages, price);
listBook.add(book);
}
resultSet.close();
statement.close();
disconnect();
return listBook;
}
public boolean deleteBook(Book book) throws SQLException {
String sql = "DELETE FROM book where id = ?";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setInt(1, book.getId());
boolean rowDeleted = statement.executeUpdate() > 0;
statement.close();
disconnect();
return rowDeleted;
}
public boolean updateBook(Book book) throws SQLException {
String sql = "UPDATE book SET title = ?, author = ?, publisher = ?, year = ?, pages = ?, price = ?";
sql += " WHERE id = ?";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setString(1, book.getTitle());
statement.setString(2, book.getAuthor());
statement.setString(3, book.getPublisher());
statement.setInt(4, book.getYear());
statement.setInt(5, book.getPages());
statement.setDouble(6, book.getPrice());
statement.setInt(7, book.getId());
boolean rowUpdated = statement.executeUpdate() > 0;
statement.close();
disconnect();
return rowUpdated;
}
public Book getBook(int id) throws SQLException {
Book book = null;
String sql = "SELECT * FROM book WHERE id = ?";
connect();
PreparedStatement statement = jdbcConnection.prepareStatement(sql);
statement.setInt(1, id);
ResultSet resultSet = statement.executeQuery();
if (resultSet.next()) {
String title = resultSet.getString("title");
String author = resultSet.getString("author");
String publisher = resultSet.getString("publisher");
int year = resultSet.getInt("year");
int pages = resultSet.getInt("pages");
double price = resultSet.getDouble("price");
book = new Book(id, title, author, publisher, year, pages, price);
}
resultSet.close();
statement.close();
return book;
}
}
```
最后,我们需要创建一个MySQL数据库,并使用以下SQL语句创建book表:
```sql
CREATE TABLE book (
id INT NOT NULL AUTO_INCREMENT,
title VARCHAR(255),
author VARCHAR(255),
publisher VARCHAR(255),
year INT,
pages INT,
price DOUBLE,
PRIMARY KEY (id)
);
```
至此,一个简单的图书信息管理系统的JAVA代码和数据库SQL语句就完成了。
相关推荐
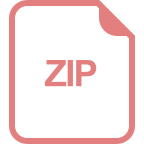














