千泽 实验十 STL应用
时间: 2023-08-29 22:13:55 浏览: 216
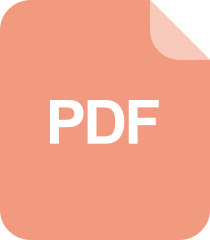
STL使用举例
好的,实验十的内容是关于STL(Standard Template Library)的应用。
STL是C++标准库中的一部分,提供了许多通用的数据结构和算法,包括容器(如vector、list、map等)、迭代器、算法(如排序、查找等)和函数对象等。STL的使用可以大大提高程序的效率和可读性。
下面是一些STL的应用实例。
1. 使用vector容器存储数据
vector是一个动态数组,可以自动调整大小以适应存储数据的需求。以下是一个例子,演示如何使用vector存储一些整数。
```c++
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<int> vec;
// 添加数据
vec.push_back(1);
vec.push_back(2);
vec.push_back(3);
// 输出数据
for (int i = 0; i < vec.size(); i++) {
cout << vec[i] << " ";
}
cout << endl;
return 0;
}
```
输出结果为:
```
1 2 3
```
2. 使用list容器存储数据
list是一个双向链表,可以在任意位置进行插入和删除操作。以下是一个例子,演示如何使用list存储一些字符串。
```c++
#include <iostream>
#include <list>
using namespace std;
int main()
{
list<string> lst;
// 添加数据
lst.push_back("hello");
lst.push_back("world");
lst.push_front("hello");
// 输出数据
for (list<string>::iterator it = lst.begin(); it != lst.end(); it++) {
cout << *it << " ";
}
cout << endl;
return 0;
}
```
输出结果为:
```
hello hello world
```
3. 使用map容器存储数据
map是一个关联数组,可以根据键值进行数据的存储和查找。以下是一个例子,演示如何使用map存储一些学生的信息。
```c++
#include <iostream>
#include <map>
using namespace std;
int main()
{
map<string, int> m;
// 添加数据
m["Tom"] = 90;
m["Jerry"] = 80;
m["Bob"] = 70;
// 查找数据
cout << "Tom's score is " << m["Tom"] << endl;
return 0;
}
```
输出结果为:
```
Tom's score is 90
```
以上是STL的一些应用实例,希望对你有帮助。
阅读全文