return { keyword: '', defaultKeyword: '', oldKeywordList: [], //历史记录数据 keywordList: [], searchTimer: null, hotWords: [], }将这个vue2写法改成vue3写法
时间: 2024-01-27 08:06:20 浏览: 12
在Vue3中,可以使用 `ref` 和 `reactive` 代替Vue2中的 `data`,同时也可以使用 `setup` 函数代替Vue2中的 `created` 和 `mounted` 钩子函数。因此,你可以将这个Vue2代码改写成如下的Vue3代码:
```
import { ref, reactive } from 'vue';
export default {
setup() {
const keyword = ref('');
const defaultKeyword = ref('');
const oldKeywordList = ref([]);
const keywordList = reactive([]);
const searchTimer = ref(null);
const hotWords = reactive([]);
return { keyword, defaultKeyword, oldKeywordList, keywordList, searchTimer, hotWords };
},
};
```
需要注意的是,Vue3中使用的是 ES6 的模块化语法,因此需要使用 `export default` 代替Vue2中的 `module.exports`。
相关问题
saveKeyword = (keyword) => { uni.getStorage({ key: 'OldKeys', success: res => { let OldKeys = JSON.parse(res.data) let findIndex = OldKeys.indexOf(keyword) if (findIndex == -1) { OldKeys.unshift(keyword) } else { OldKeys.splice(findIndex, 1) OldKeys.unshift(keyword) } //最多10个纪录 OldKeys.length > 10 && OldKeys.pop() uni.setStorage({ key: 'OldKeys', data: JSON.stringify(OldKeys) }) oldKeywordList = OldKeys //更新历史搜索 }, fail: e => { var OldKeys = [keyword] uni.setStorage({ key: 'OldKeys', data: JSON.stringify(OldKeys) }) oldKeywordList = OldKeys //更新历史搜索 } })换成vue3写法
在 Vue 3 中,可以使用 `localStorage` API 来进行本地存储。可以使用 `reactive` 和 `computed` 来实现响应式数据和计算属性,将方法定义在 `setup` 函数中。下面是修改后的代码:
```
<template>
<div>
<!-- 其他模板内容 -->
</div>
</template>
<script>
import { reactive, computed, onMounted } from 'vue'
export default {
setup() {
// 定义响应式数据
const state = reactive({
keywordList: [],
maxKeywordCount: 10,
defaultKeyword: 'default',
keyword: ''
})
// 计算属性
const oldKeywordList = computed(() => {
return state.keywordList.slice(1, state.maxKeywordCount + 1)
})
// 定义方法
function saveKeyword(keyword) {
let OldKeys = JSON.parse(localStorage.getItem('OldKeys')) || []
let findIndex = OldKeys.indexOf(keyword)
if (findIndex == -1) {
OldKeys.unshift(keyword)
} else {
OldKeys.splice(findIndex, 1)
OldKeys.unshift(keyword)
}
// 最多 maxKeywordCount 个记录
OldKeys.length > state.maxKeywordCount && OldKeys.pop()
localStorage.setItem('OldKeys', JSON.stringify(OldKeys))
state.keywordList = OldKeys // 更新历史搜索
}
// 生命周期函数
onMounted(() => {
// 从本地存储中获取历史搜索列表
let OldKeys = JSON.parse(localStorage.getItem('OldKeys')) || []
state.keywordList = OldKeys
})
// 返回响应式数据和方法
return { state, oldKeywordList, saveKeyword }
}
}
</script>
```
在 `setup` 函数中,通过 `reactive` 定义了响应式数据 `state`,包含了 `keywordList`、`maxKeywordCount`、`defaultKeyword` 和 `keyword` 四个属性。使用 `computed` 定义了响应式计算属性 `oldKeywordList`,用于计算最近搜索的关键字列表。定义了方法 `saveKeyword`,用于保存关键字到本地存储中,并更新历史搜索列表。使用 `onMounted` 生命周期函数,从本地存储中获取历史搜索列表并更新响应式数据 `state` 中的 `keywordList` 属性。最后,在返回的对象中返回了 `state`、`oldKeywordList` 和 `saveKeyword` 三个属性。
<WMS_Capabilities xmlns="http://www.opengis.net/wms" xmlns:xlink="http://www.w3.org/1999/xlink" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" version="1.3.0" updateSequence="7578" xsi:schemaLocation="http://www.opengis.net/wms http://172.16.11.48:8180/geoserver/schemas/wms/1.3.0/capabilities_1_3_0.xsd"> <Service> <Name>WMS</Name> <Title>My GeoServer WMS</Title> <Abstract> This is a description of your Web Map Server. </Abstract> <KeywordList> <Keyword>WFS</Keyword> <Keyword>WMS</Keyword> <Keyword>GEOSERVER</Keyword> </KeywordList> <OnlineResource xlink:type="simple" xlink:href="http://geoserver.org"/> <ContactInformation> <ContactPersonPrimary> <ContactPerson/> <ContactOrganization/> </ContactPersonPrimary> <ContactPosition/> <ContactAddress> <AddressType/> <Address/> <City/> <StateOrProvince/> <PostCode/> <Country/> </ContactAddress> <ContactVoiceTelephone/> <ContactFacsimileTelephone/> <ContactElectronicMailAddress/> </ContactInformation> <Fees>NONE</Fees> <AccessConstraints>NONE</AccessConstraints> </Service>转json
根据您提供的信息,您似乎正在尝试将一个 WMS_Capabilities 文件转换为 JSON 格式。由于这是一个 XML 文件,并非直接的 JSON 格式,您需要先将其转换为 JSON。
您可以使用适当的库或解析器来处理 XML 文件,并将其转换为 JSON 格式。以下是一个使用 JavaScript 的示例代码:
```javascript
const xmlString = `<!-- 在这里插入您提供的 XML 字符串 -->`;
// 使用 DOMParser 解析 XML
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlString, "text/xml");
// 使用 XML2JS 将 XML 转换为 JSON
const xml2js = require("xml2js");
const parser = new xml2js.Parser();
parser.parseString(xmlDoc, (err, result) => {
if (err) {
console.error("XML 转换成 JSON 出错:", err);
return;
}
const json = JSON.stringify(result);
console.log(json);
});
```
请注意,上述示例中使用了 DOMParser 和 xml2js 库来解析 XML 并将其转换为 JSON。您可能需要根据您的具体使用环境和语言选择相应的库。
希望这对您有所帮助!如果您有任何进一步的问题,请随时提问。
相关推荐
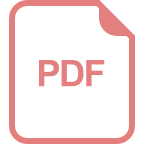




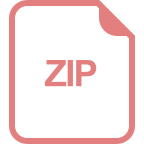
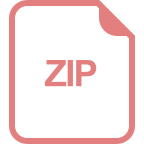
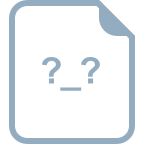
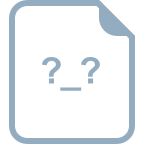
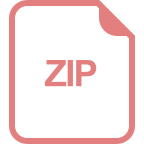
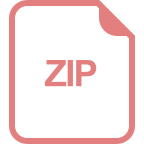
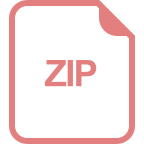
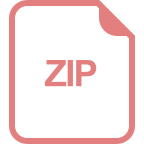
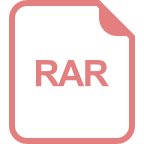