rbf神经网络vc程序代码
时间: 2023-09-17 19:04:49 浏览: 108
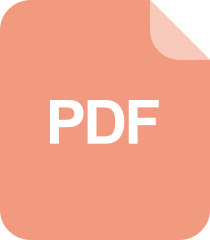
RBF神经网络详细代码

RBF(Radial Basis Function)神经网络是一种常用的人工神经网络模型之一。下面是一个简单的RBF神经网络的VC程序代码示例:
```
#include <iostream>
#include <cmath>
#include <vector>
// 定义RBF神经网络的数据结构
struct RBFNetwork {
std::vector<double> centers; // 中心点坐标
std::vector<double> weights; // 权重
double sigma; // RBF函数的标准差
};
// RBF神经网络的训练函数
void train(RBFNetwork& network, const std::vector<double>& input, double target) {
// 1. 计算输入样本与中心点之间的距离
std::vector<double> distances;
for (int i = 0; i < network.centers.size(); ++i) {
double distance = 0;
for (int j = 0; j < input.size(); ++j) {
distance += pow(input[j] - network.centers[i], 2);
}
distance = sqrt(distance);
distances.push_back(distance);
}
// 2. 使用RBF函数计算输出值
double output = 0;
for (int i = 0; i < distances.size(); ++i) {
output += network.weights[i] * exp(-pow(distances[i], 2) / (2 * pow(network.sigma, 2)));
}
// 3. 更新权重
double error = target - output;
for (int i = 0; i < distances.size(); ++i) {
network.weights[i] += error * exp(-pow(distances[i], 2) / (2 * pow(network.sigma, 2)));
}
}
int main() {
// 创建一个RBF神经网络对象
RBFNetwork network;
// 设置网络参数
network.centers = {1.0, 2.0, 3.0}; // 设置中心点坐标
network.weights = {0.5, -0.5, 1.0}; // 设置权重
network.sigma = 0.5; // 设置RBF函数的标准差
// 定义输入样本和目标输出
std::vector<double> input = {0.5, 1.5, 2.5};
double target = 1.5;
// 使用训练函数进行训练
train(network, input, target);
// 打印训练后的权重值
for (int i = 0; i < network.weights.size(); ++i) {
std::cout << "Weight " << i << ": " << network.weights[i] << std::endl;
}
return 0;
}
```
以上代码示例中,首先定义了一个RBF神经网络的数据结构,包含了中心点坐标、权重和RBF函数的标准差等参数。然后,定义了训练函数`train`,实现了RBF神经网络的训练过程。在`main`函数中,通过设置网络参数、输入样本和目标输出,使用训练函数进行训练,并打印训练后的权重值。
阅读全文
相关推荐
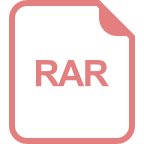
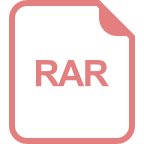
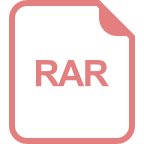
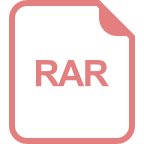
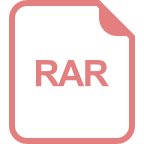
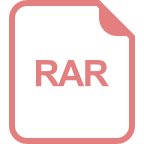
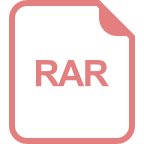
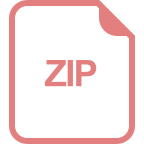
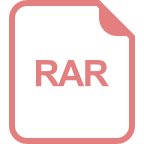
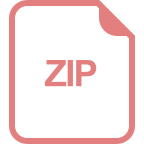
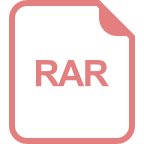