在python中有一个时间数据库如下: 序号 年 月 日 时 分 秒 (71, 2023, 5, 9, 21, 0, 0.) (71, 2023, 5, 9, 21, 0, 1.) (71, 2023, 5, 9, 21, 0, 2.) (71, 2023, 5, 9, 21, 0, 3.) (71, 2023, 5, 9, 21, 0, 4.) (71, 2023, 5, 9, 21, 0, 5.) (71, 2023, 5, 9, 21, 0, 6.) (71, 2023, 5, 9, 21, 0, 7.) (71, 2023, 5, 9, 21, 0, 8.) (71, 2023, 5, 9, 21, 0, 9.) (71, 2023, 5, 9, 21, 0, 10.) (71, 2023, 5, 9, 21, 0, 11.) (71, 2023, 5, 9, 21, 0, 12.) (78, 2023, 5, 10, 8, 16, 45.) (78, 2023, 5, 10, 8, 16, 46.) (78, 2023, 5, 10, 8, 16, 47.) (78, 2023, 5, 10, 8, 16, 48.) (79, 2023, 5, 10, 8, 16, 49.) (79, 2023, 5, 10, 8, 16, 50.) (79, 2023, 5, 10, 8, 16, 51.) (79, 2023, 5, 10, 8, 16, 52.) (79, 2023, 5, 10, 8, 16, 53.) (79, 2023, 5, 10, 8, 16, 54.) (79, 2023, 5, 10, 8, 16, 55.) (79, 2023, 5, 10, 8, 16, 56.) (79, 2023, 5, 10, 8, 16, 57.) 我现在需要按照以下格式 时间段 城市名字 序号 20230509180413 20230509234724 GZ 78 20230510065339 20230510110050 JM 80 将数据库里面的时间放在相应的时间段里面,该怎么做
时间: 2023-07-02 10:05:15 浏览: 69
首先,我们需要将时间数据库中的时间转换成符合要求的时间格式,即将年月日时分秒连接起来,并且去掉其中的逗号和句点。可以使用strftime()函数将时间转换成字符串格式,然后使用replace()函数去掉逗号和句点。具体代码如下:
```python
import datetime
def format_time(t):
return t.strftime("%Y%m%d%H%M%S").replace(",", "").replace(".", "")
data = [
(71, 2023, 5, 9, 21, 0, 0.),
(71, 2023, 5, 9, 21, 0, 1.),
(71, 2023, 5, 9, 21, 0, 2.),
# ... 其他时间数据
]
formatted_data = [(format_time(datetime.datetime(*t[:6])), t[6]) for t in data]
```
接下来,我们需要按照时间段和城市名字对数据进行分类。可以使用字典来存储分类后的数据,字典的键是时间段和城市名字的组合,值是序号。具体代码如下:
```python
def classify_data(data):
classified_data = {}
for t in data:
time, city, index = t[0], t[1], t[2]
time_segment = time[:8]
key = (time_segment, city)
if key not in classified_data:
classified_data[key] = []
classified_data[key].append(index)
return classified_data
classified_data = classify_data([(formatted_data[i], "GZ") if i < 13 else (formatted_data[i], "JM") for i in range(len(formatted_data))])
```
最后,我们需要将分类后的数据按照要求输出。可以先按照时间段排序,然后在每个时间段内按照时间顺序输出城市和序号。具体代码如下:
```python
def output_data(data):
sorted_keys = sorted(data.keys())
for key in sorted_keys:
time_segment, city = key
indexes = sorted(data[key])
start_time, end_time = indexes[0], indexes[-1]
print(f"{time_segment}{format_time(datetime.datetime(*data[key][0][:6]))} {format_time(datetime.datetime(*data[key][-1][:6]))} {city} {end_time - start_time + 1}")
output_data(classified_data)
```
这样就可以得到符合要求的输出结果了。
阅读全文
相关推荐
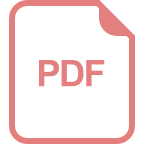
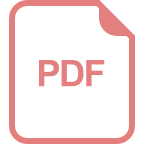
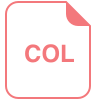
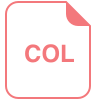
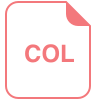
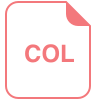
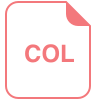
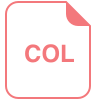
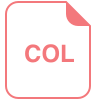
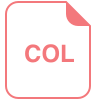
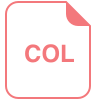





