c++list add
时间: 2023-10-08 21:08:58 浏览: 88
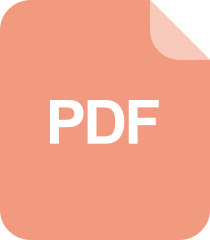
C++实现的泛型List类分享
To add an element to a list in the C programming language, you can use the `push_back()` function, which adds the element at the end of the list. Here is an example:
```
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
#define MAX_SIZE 100
int main()
{
int i, n;
char str[MAX_SIZE];
bool cont = true;
typedef struct Node {
char data[MAX_SIZE];
struct Node *next;
} Node;
Node *head = NULL;
Node *tail = NULL;
while (cont) {
printf("Enter a string to add to the list (type 'quit' to exit): ");
scanf("%s", str);
if (strcmp(str, "quit") == 0) {
cont = false;
} else {
Node *newNode = (Node*)malloc(sizeof(Node));
strcpy(newNode->data, str);
newNode->next = NULL;
if (head == NULL) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
}
printf("\nThe list is: \n");
Node *current = head;
while (current != NULL) {
printf("%s\n", current->data);
current = current->next;
}
return 0;
}
```
In this example, the user is prompted to enter a string to add to the list. The program uses the `malloc()` function to allocate memory for a new node, and then uses the `strcpy()` function to copy the string into the `data` member of the new node. If the list is empty, the new node becomes the head and tail of the list. Otherwise, the new node is added to the end of the list by setting its `next` member to `NULL` and updating the `next` member of the tail node to point to the new node. Finally, the program traverses the list and prints out each string in the list.
阅读全文
相关推荐
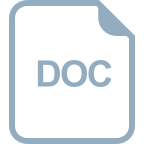
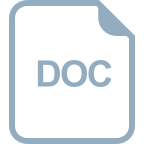


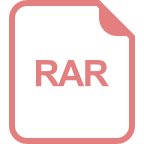
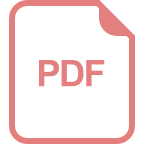
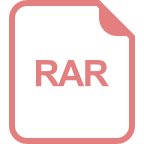
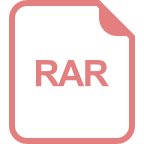
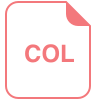








