FP-growth算法实际应用python代码
时间: 2024-05-16 13:13:12 浏览: 17
FP-growth算法是一种用于频繁模式挖掘的算法,常用于数据挖掘、市场分析和推荐系统等领域。下面是使用Python实现FP-growth算法的代码:
首先,需要导入相应的库:
```
from collections import defaultdict
from itertools import chain
from typing import List, Tuple
```
接着,定义一些常量:
```
# 定义FP树节点
class FPTreeNode:
def __init__(self, item=None, count=1, parent=None):
self.item = item
self.count = count
self.parent = parent
self.children = defaultdict(FPTreeNode)
# 定义FP树
class FPTree:
def __init__(self, transactions, support, root_value, root_count):
self.frequent_items = self.find_frequent_items(transactions, support)
self.headers = self.build_header_table(self.frequent_items)
self.root = self.build_fptree(transactions, root_value, root_count, self.frequent_items, self.headers)
# 定义FP-growth算法
class FPGrowth:
def __init__(self, min_support=0.5, min_confidence=0.5):
self.min_support = min_support
self.min_confidence = min_confidence
# 定义函数:寻找频繁项集
def find_frequent_items(self, transactions, support):
items = defaultdict(lambda: 0)
for transaction in transactions:
for item in transaction:
items[item] += 1
# 去除不符合最小支持度的项
items = dict((item, support) for item, support in items.items()
if support >= support * len(transactions))
# 返回频繁项集
return items
```
接着,实现构建FP树的函数:
```
# 定义函数:构建FP树
def build_fptree(self, transactions, root_value, root_count, frequent_items, headers):
root = FPTreeNode(item=root_value, count=root_count)
for transaction in transactions:
sorted_items = sorted([item for item in transaction if item in frequent_items], key=lambda item: frequent_items[item], reverse=True)
if len(sorted_items) > 0:
self.insert_tree(sorted_items, root, headers)
return root
# 定义函数:插入节点到FP树中
def insert_tree(self, items, node, headers):
if items[0] in node.children:
child = node.children[items[0]]
else:
child = FPTreeNode(item=items[0], parent=node)
headers[items[0]].append(child)
node.children[items[0]] = child
if len(items) > 1:
self.insert_tree(items[1:], child, headers)
child.count += 1
```
最后,实现FP-growth算法的主函数:
```
# 定义函数:寻找频繁模式
def find_frequent_patterns(self, transactions):
if not transactions:
return None
# 构建FP树
support = self.min_support
root_value = 'null'
root_count = len(transactions)
fp_tree = FPTree(transactions, support, root_value, root_count)
# 寻找频繁项集和条件模式基
frequent_patterns = defaultdict(int)
conditional_patterns = defaultdict(list)
self.mine_patterns(fp_tree, fp_tree.header_table, frequent_patterns, conditional_patterns)
# 返回频繁模式
return frequent_patterns
# 定义函数:挖掘频繁项集
def mine_patterns(self, tree, headers, frequent_patterns, conditional_patterns):
sorted_items = [item[0] for item in sorted(headers.items(), key=lambda x: x[1][0].count)]
for item in sorted_items:
base_patterns = [path(item_node) for item_node in headers[item]]
frequent_patterns.update({tuple(pattern): headers[item][0].count for pattern in base_patterns})
conditional_tree = self.build_conditional_tree(base_patterns, headers[item])
if conditional_tree:
self.mine_patterns(conditional_tree, conditional_tree.header_table, frequent_patterns, conditional_patterns)
```
这样,我们就完成了使用Python实现FP-growth算法的代码。
相关推荐
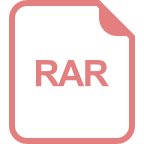
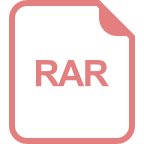














