C 语言遍历文件夹下的文件
时间: 2023-09-07 12:14:23 浏览: 73
在 C 语言中,可以使用 dirent.h 头文件中的函数遍历文件夹下的文件。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <dirent.h>
int main() {
DIR *dir;
struct dirent *ent;
if ((dir = opendir(".")) != NULL) {
while ((ent = readdir(dir)) != NULL) {
printf("%s\n", ent->d_name);
}
closedir(dir);
} else {
perror("Unable to open directory");
return 1;
}
return 0;
}
```
上述程序中,首先打开当前目录("."),遍历目录下的所有文件和子目录,并输出文件名。在实际应用中,可以根据需求修改目录路径和输出方式等。
另外,需要注意的是,dirent.h 头文件中的函数在 Windows 平台和 Linux 平台下的表现可能不同,需要根据实际情况进行修改。
相关问题
c 语言遍历文件夹下的以”.log“结尾的文件
好的,以下是一个遍历指定目录下的所有文件,并只处理以“.log”结尾的文件的C语言示例代码,您可以参考一下:
```c
#include <stdio.h>
#include <dirent.h>
#include <string.h>
#include <stdlib.h>
#include <sys/stat.h>
int process_log_file(char *file_path) {
// 处理.log文件的代码
return 0;
}
int traverse_dir(char *dir_path) {
DIR *dir;
struct dirent *entry;
struct stat statbuf;
if ((dir = opendir(dir_path)) == NULL) {
perror("Error opening directory");
return -1;
}
while ((entry = readdir(dir)) != NULL) {
char full_path[1024];
sprintf(full_path, "%s/%s", dir_path, entry->d_name);
if (stat(full_path, &statbuf) == -1) {
continue;
}
if (S_ISDIR(statbuf.st_mode)) {
if (strcmp(".", entry->d_name) == 0 || strcmp("..", entry->d_name) == 0) {
continue;
}
traverse_dir(full_path);
} else {
if (strcmp(".log", strrchr(entry->d_name, '.')) == 0) {
process_log_file(full_path);
}
}
}
closedir(dir);
return 0;
}
```
您可以将要处理的代码替换掉 `process_log_file` 函数中的占位符,然后将要遍历的目录路径传递给 `traverse_dir` 函数即可。
VBA 遍历文件夹下所有文件
VBA(Visual Basic for Applications)是微软Office应用程序(如Excel、Word等)内置的一种宏语言,可以用来自动化任务。遍历文件夹下的所有文件通常通过`Folder`对象及其相关方法来完成。以下是一个简单的例子,展示如何在VBA中遍历指定文件夹并列出其内容:
```vba
Sub ListFilesInFolder()
Dim objFSO As Object ' FileSystemObject用于操作文件系统
Set objFSO = CreateObject("Scripting.FileSystemObject")
Dim objFolder As Object ' 代表特定文件夹
Dim objFile As Object ' 代表单个文件
Dim strFolderPath As String ' 要遍历的文件夹路径
' 替换 "C:\YourFolderPath" 为实际的文件夹路径
strFolderPath = "C:\YourFolderPath"
On Error Resume Next ' 捕获可能出现的错误
Set objFolder = objFSO.GetFolder(strFolderPath)
If Not objFolder Is Nothing Then ' 如果文件夹存在
For Each objFile In objFolder.Files
Debug.Print objFile.Name ' 输出文件名
Next objFile
If Err.Number <> 0 Then ' 如果有错误发生
MsgBox "Error occurred while listing files: " & Err.Description
Err.Clear ' 清除错误
End If
Else
MsgBox "Folder not found at " & strFolderPath
End If
Set objFile = Nothing
Set objFolder = Nothing
Set objFSO = Nothing
End Sub
```
在这个脚本中,我们首先创建了一个`FileSystemObject`实例,然后获取了指定路径的文件夹。接着使用`Files`集合来迭代文件夹中的每个文件,并打印出它们的名称。
阅读全文
相关推荐
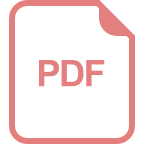
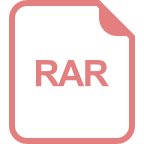
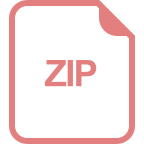
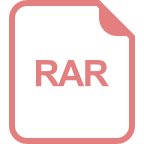
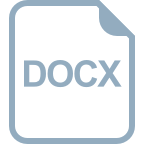
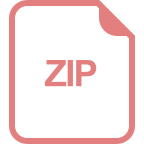
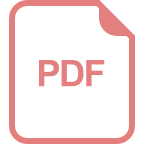



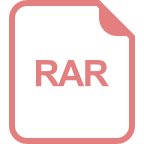
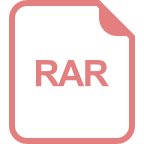
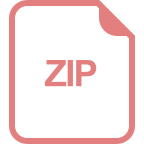
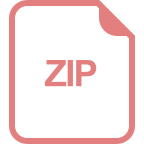