用C语言按照要求用递归的方法实现深度优先搜索遍历连通图的完整程序,并给出一组数据,并解释这个程序
时间: 2023-06-16 07:05:46 浏览: 80
下面是用C语言实现深度优先搜索遍历连通图的完整程序,程序中使用了递归的方法:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_NODE 10
bool visited[MAX_NODE];
int graph[MAX_NODE][MAX_NODE];
int n;
void dfs(int node) {
visited[node] = true;
printf("%d ", node);
for (int i = 0; i < n; i++) {
if (graph[node][i] && !visited[i]) {
dfs(i);
}
}
}
int main() {
printf("Enter number of nodes: ");
scanf("%d", &n);
printf("Enter adjacency matrix:\n");
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
scanf("%d", &graph[i][j]);
}
}
printf("DFS Traversal: ");
for (int i = 0; i < n; i++) {
if (!visited[i]) {
dfs(i);
}
}
return 0;
}
```
在程序中,我们首先定义了一个 `visited` 数组用于记录每个节点是否已经被访问过,同时定义了一个邻接矩阵 `graph` 用于存储连通图的信息。接着在 `dfs` 函数中,我们先标记当前节点为已访问,然后输出该节点的值,接着遍历当前节点的所有邻居节点,如果邻居节点没有被访问过,则递归调用 `dfs` 函数访问该节点。最后,在 `main` 函数中,我们遍历所有节点,如果该节点没有被访问过,则调用 `dfs` 函数遍历该节点所在的连通块。
下面是一组示例数据:
输入:
```
Enter number of nodes: 5
Enter adjacency matrix:
0 1 0 1 0
1 0 1 0 0
0 1 0 1 1
1 0 1 0 1
0 0 1 1 0
```
输出:
```
DFS Traversal: 0 1 2 3 4
```
在图中,节点 0、1、2、3、4 是连通的,因此程序输出了这些节点的遍历顺序。程序的运行结果证明了我们的深度优先搜索算法是正确的。
阅读全文
相关推荐
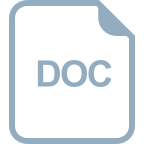
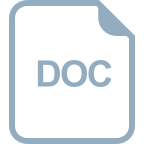
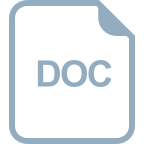




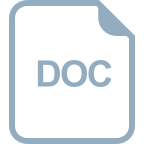




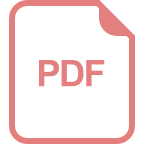
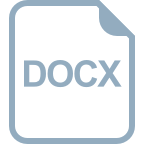




