用C语言一个连通图采用邻接表作为存储结构,设计一个算法,实现从顶点v出发,对图进行深度优先遍历的非递归过程。
时间: 2024-01-15 11:43:43 浏览: 54
好的,我会帮您解决这个问题。以下是基于邻接表存储结构的深度优先遍历非递归算法的C语言实现代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100 // 最大顶点个数
typedef struct ArcNode { // 边表结点
int adjvex; // 该弧所指向的顶点的位置
struct ArcNode* next; // 指向下一条弧的指针
} ArcNode;
typedef struct VNode { // 顶点表结点
int data; // 顶点数据
ArcNode* firstarc; // 指向第一条依附该顶点的弧的指针
} VNode, AdjList[MAX_VERTEX_NUM];
typedef struct {
AdjList vertices; // 邻接表
int vexnum; // 顶点数
int arcnum; // 边数
} ALGraph;
typedef struct {
int data[MAX_VERTEX_NUM]; // 存储栈元素
int top; // 栈顶指针
} Stack;
void InitStack(Stack* S) { // 初始化栈
S->top = -1;
}
int StackEmpty(Stack* S) { // 判断栈是否为空
return S->top == -1;
}
void Push(Stack* S, int e) { // 进栈
if (S->top == MAX_VERTEX_NUM - 1) {
printf("Error: Stack is full!\n");
return;
}
S->data[++S->top] = e;
}
void Pop(Stack* S, int* e) { // 出栈
if (StackEmpty(S)) {
printf("Error: Stack is empty!\n");
return;
}
*e = S->data[S->top--];
}
int Visit[MAX_VERTEX_NUM]; // 访问标志数组,全局变量
void DFS(ALGraph* G, int v) { // 非递归深度优先遍历算法
Stack S;
InitStack(&S);
Push(&S, v);
while (!StackEmpty(&S)) {
int u;
Pop(&S, &u);
if (!Visit[u]) {
Visit[u] = 1;
printf("%d ", G->vertices[u].data); // 访问顶点u
ArcNode* p = G->vertices[u].firstarc;
while (p) {
if (!Visit[p->adjvex]) {
Push(&S, p->adjvex); // 将未访问过的邻接顶点进栈
}
p = p->next;
}
}
}
}
int main() {
ALGraph G;
int i, j, k;
printf("Enter the number of vertices and arcs of the graph (vexnum arcnum): ");
scanf("%d %d", &G.vexnum, &G.arcnum);
printf("Enter the data of each vertex: ");
for (i = 0; i < G.vexnum; i++) {
scanf("%d", &G.vertices[i].data);
G.vertices[i].firstarc = NULL;
}
printf("Enter the head vertex and tail vertex of each arc (start from 0):\n");
for (k = 0; k < G.arcnum; k++) {
scanf("%d %d", &i, &j);
ArcNode* e = (ArcNode*) malloc(sizeof(ArcNode));
e->adjvex = j;
e->next = G.vertices[i].firstarc;
G.vertices[i].firstarc = e;
}
printf("The depth first search sequence of the graph starting from vertex 0 is: ");
for (i = 0; i < G.vexnum; i++) {
Visit[i] = 0; // 初始化访问标志数组
}
DFS(&G, 0); // 从顶点0开始深度优先遍历
printf("\n");
return 0;
}
```
实现原理是使用一个栈来存储待访问的顶点,从起始顶点开始进栈,不断弹出栈顶的顶点,访问它并将未被访问过的邻接顶点进栈,直到栈为空为止。为了避免重复访问已经访问过的顶点,使用一个访问标志数组Visit来记录每个顶点的访问状态。
相关推荐
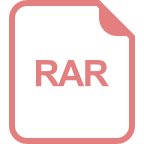
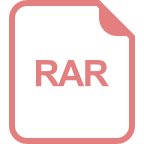
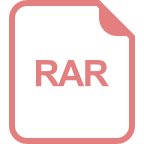
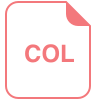
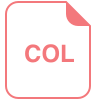
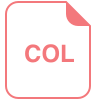
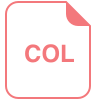
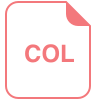









