def __init__(self, model_path): self.model = torch.load(model_path, map_location=torch.device('cpu')) self.model.eval()
时间: 2024-05-21 07:13:33 浏览: 141
这段代码是ChitGPT模型的初始化函数。它接受一个模型路径作为参数,使用PyTorch的torch.load函数将模型加载到内存中,并将模型设置为eval模式,以便在推理时使用。map_location=torch.device('cpu')指定了将模型加载到CPU上,这是因为如果没有GPU可以使用,程序就会崩溃。
相关问题
import torchimport cv2import numpy as npfrom models.experimental import attempt_loadfrom utils.general import non_max_suppressionclass YoloV5Detector: def __init__(self, model_path, conf_thresh=0.25, iou_thresh=0.45): self.conf_thresh = conf_thresh self.iou_thresh = iou_thresh self.device = torch.device("cuda" if torch.cuda.is_available() else "cpu") self.model = attempt_load(model_path, map_location=self.device) self.model.eval() def detect(self, image_path): img = cv2.imread(image_path) img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) img = img.transpose(2, 0, 1) img = np.ascontiguousarray(img) img = torch.from_numpy(img).to(self.device).float() / 255.0 # Run inference with torch.no_grad(): results = self.model(img, size=img.shape[-2:]) results = non_max_suppression(results, conf_thres=self.conf_thresh, iou_thres=self.iou_thresh) return results
这是一个使用 YOLOv5 模型进行目标检测的 Python 代码。该类 YoloV5Detector 包含了模型加载、图片预处理和推理的功能。具体来说,会使用 OpenCV 库读取图片,将其转换为 RGB 格式,然后转换为 PyTorch 的 Tensor 格式,并将其送入 YOLOv5 模型中进行推理。最后,使用非极大值抑制算法(NMS)筛选出检测出来的物体,并返回结果。其中,conf_thresh 和 iou_thresh 分别表示置信度和重叠阈值,可以通过调整这两个参数来控制检测结果的准确率和召回率。
def __init__(self, dataset='mydata'): self.config = BertConfig(dataset) self.model = MyBertModel(self.config).to('cpu') self.model.load_state_dict(torch.load(self.config.save_path, map_location='cpu'))
这段代码是一个 Python 类的初始化函数,其中:
- `dataset` 是一个可选参数,默认值为 `'mydata'`;
- `BertConfig(dataset)` 用于创建一个 BERT 模型的配置对象,该对象的参数由 `dataset` 决定;
- `MyBertModel(self.config)` 用于创建一个 BERT 模型,该模型的具体结构由 `MyBertModel` 类定义;
- `.to('cpu')` 将模型移动到 CPU 上进行计算;
- `torch.load(self.config.save_path, map_location='cpu')` 从本地加载 BERT 模型的参数,`self.config.save_path` 是模型参数的保存路径,`map_location='cpu'` 表示将模型参数加载到 CPU 上进行计算。
综上,这段代码的作用是创建一个 BERT 模型,并加载之前训练好的模型参数,以便进行后续的预测或者推理。
阅读全文
相关推荐
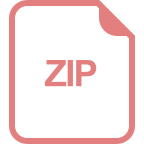
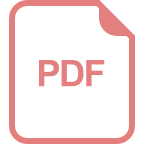


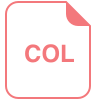
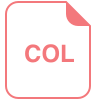
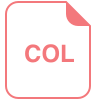
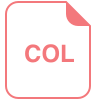
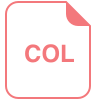



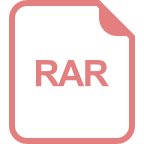
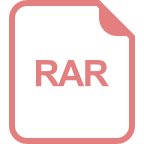