简易图书管理系统python
时间: 2023-09-12 18:13:26 浏览: 78
下是一个简单的图书管理系统的Python代码示例:
```python
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def remove_book(self, book):
self.books.remove(book)
def search_book(self, title):
for book in self.books:
if book.title == title:
return book
return None
def main():
library = Library()
book1 = Book("The Catcher in the Rye", "J.D. Salinger", "9780316769174")
book2 = Book("To Kill a Mockingbird", "Harper Lee", "9780061120084")
book3 = Book("1984", "George Orwell", "9780451524935")
library.add_book(book1)
library.add_book(book2)
library.add_book(book3)
print("Welcome to the library!")
while True:
print("1. Search for a book")
print("2. Add a book")
print("3. Remove a book")
print("4. Quit")
choice = input("Enter your choice: ")
if choice == "1":
title = input("Enter the title of the book: ")
book = library.search_book(title)
if book:
print(f"{book.title} by {book.author} (ISBN: {book.isbn})")
else:
print(f"{title} not found in the library.")
elif choice == "2":
title = input("Enter the title of the book: ")
author = input("Enter the author of the book: ")
isbn = input("Enter the ISBN of the book: ")
book = Book(title, author, isbn)
library.add_book(book)
print(f"{title} added to the library.")
elif choice == "3":
title = input("Enter the title of the book: ")
book = library.search_book(title)
if book:
library.remove_book(book)
print(f"{book.title} removed from the library.")
else:
print(f"{title} not found in the library.")
elif choice == "4":
print("Goodbye!")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
```
这个程序包括两个类,`Book` 和 `Library`。`Book` 表示一本书,包括标题、作者和 ISBN 号。`Library` 表示图书馆,包括一个 `books` 列表,可以添加、删除和搜索图书。
在 `main()` 函数中,首先创建一个空的图书馆,然后添加三本书。接下来,使用一个无限循环让用户选择要做什么。如果用户选择搜索书籍,则输入书名并在图书馆中搜索。如果用户选择添加书籍,则输入书名、作者和 ISBN 号,并将新书添加到图书馆中。如果用户选择删除书籍,则输入书名并在图书馆中删除。如果用户选择退出,则退出程序。
阅读全文
相关推荐
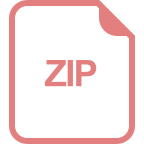
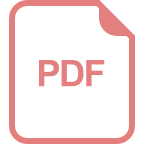
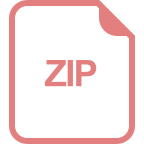















