this.STATES = { 'LOADING': 'loading', 'PLAYING': 'playing', 'READY': 'ready', 'ENDED': 'ended', 'RESETTING': 'resetting' }; this.blocks = []; this.state = this.STATES.LOADING; this.stage = new Stage(); this.mainContainer = document.getElementById('container'); this.scoreContainer = document.getElementById('score'); this.startButton = document.getElementById('start-button'); this.instructions = document.getElementById('instructions'); this.scoreContainer.innerHTML = '0'; this.newBlocks = new THREE.Group(); this.placedBlocks = new THREE.Group(); this.choppedBlocks = new THREE.Group(); this.stage.add(this.newBlocks); this.stage.add(this.placedBlocks); this.stage.add(this.choppedBlocks); this.addBlock(); this.tick(); this.updateState(this.STATES.READY);
时间: 2024-03-31 10:37:29 浏览: 231
这段代码看起来是某个游戏的初始化过程,其中定义了一些游戏状态的常量,并初始化了一些变量和DOM元素。其中,this.blocks数组看起来是用于存储游戏中的方块,this.stage是一个THREE.js的场景对象,分别添加了三个THREE.Group对象,用于存放不同状态的方块。最后,调用了addBlock方法和tick方法,以及设置游戏状态为READY状态。可以看出,这个代码片段是用于初始化游戏环境的。
相关问题
帮我优化这段代码if(this.RB > 0 && this.isbuy == 0){ this.maskShow = true; this.canSee = false; }else{ this.maskShow = false; } if(this.player && this.player.ended()&& (this.RB==0||this.isbuy==1)){ this.canSee = true } if(this.player){ this.player.pause(); }
可以对这段代码进行一些优化,可以将条件判断简化并且合并一些逻辑。优化后的代码如下:
```javascript
if (this.RB > 0 && this.isbuy == 0) {
this.maskShow = true;
this.canSee = false;
} else {
this.maskShow = false;
if (this.player && this.player.ended() && (this.RB == 0 || this.isbuy == 1)) {
this.canSee = true;
}
}
if (this.player) {
this.player.pause();
}
```
这样做的好处是减少了重复的条件判断,并且将相关的操作放在一起,使代码更加清晰和易读。
class AudioPlayer { constructor(src, loop = false) { this.audio = new Audio(src); this.audio.loop = loop; this.isPlaying = false; this.hasError = false; this.logError(); } play() { if (!this.hasError) { this.audio.play(); this.isPlaying = true; } } pause() { if (!this.hasError) { this.audio.pause(); this.isPlaying = false; } } stop() { if (!this.hasError) { this.audio.pause(); this.audio.currentTime = 0; this.isPlaying = false; } } logError() { this.audio.onerror = () => { console.error("Your browser does not support audio playback."); this.hasError = true; }; } } 优化上述代码,增加在播放时指定播放次数的属性
可以在构造函数中增加一个参数 `playCount`,默认为1,表示播放次数。同时,可以在 `play()` 方法中添加逻辑,当播放次数大于0时才播放,并且每次播放完成后将播放次数减1。当播放次数为0时,自动停止播放。
优化后的代码如下:
```javascript
class AudioPlayer {
constructor(src, loop = false, playCount = 1) {
this.audio = new Audio(src);
this.audio.loop = loop;
this.isPlaying = false;
this.hasError = false;
this.playCount = playCount;
this.logError();
this.audio.addEventListener("ended", () => {
if (this.playCount > 0) {
this.playCount--;
if (this.playCount === 0) {
this.stop();
} else {
this.play();
}
}
});
}
play() {
if (!this.hasError && this.playCount > 0) {
this.audio.play();
this.isPlaying = true;
}
}
pause() {
if (!this.hasError) {
this.audio.pause();
this.isPlaying = false;
}
}
stop() {
if (!this.hasError) {
this.audio.pause();
this.audio.currentTime = 0;
this.isPlaying = false;
}
}
logError() {
this.audio.onerror = () => {
console.error("Your browser does not support audio playback.");
this.hasError = true;
};
}
}
```
使用示例:
```javascript
const player = new AudioPlayer("music.mp3", true, 3); // 播放3次
player.play(); // 开始播放
```
阅读全文
相关推荐
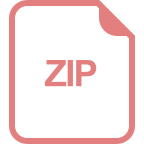
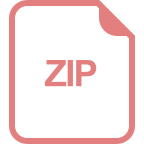
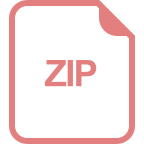










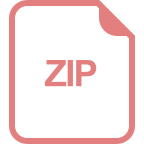