首页设计一个词典类Dic,每个单词包括英文单词及对应的中文含义,并有一个英汉翻译成员函数,通过查词典的方式将英语翻译成对应的汉语。 思路: 字典项类DicItem包括EngLish(英语单词)、Chinese(对应中文含义)数据成员,字典类包括一个字典项类的列表,包含Add()(添加单词)和trans(英汉翻译)成员函数。
设计一个词典类Dic,每个单词包括英文单词及对应的中文含义,并有一个英汉翻译成员函数,通过查词典的方式将英语翻译成对应的汉语。 思路: 字典项类DicItem包括EngLish(英语单词)、Chinese(对应中文含义)数据成员,字典类包括一个字典项类的列表,包含Add()(添加单词)和trans(英汉翻译)成员函数。
时间: 2023-06-12 19:08:30 浏览: 348
以下是 Python 代码实现:
```python
class DicItem:
def __init__(self, english, chinese):
self.english = english
self.chinese = chinese
class Dic:
def __init__(self):
self.items = []
def add(self, english, chinese):
item = DicItem(english, chinese)
self.items.append(item)
def trans(self, english):
for item in self.items:
if item.english == english:
return item.chinese
return "未找到该单词的中文含义"
```
使用示例:
```python
d = Dic()
d.add("apple", "苹果")
d.add("banana", "香蕉")
d.add("orange", "橙子")
print(d.trans("apple")) # 输出:苹果
print(d.trans("pear")) # 输出:未找到该单词的中文含义
```
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
大家在看
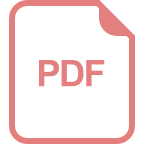
MS入门教程
MS入门教程,简易教程,操作界面,画图建模等入门内容。
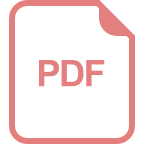
一种新型三自由度交直流混合磁轴承原理及有限元分析
研究了一种新颖的永磁偏磁三自由度交直流混合磁轴承。轴向悬浮力控制采用直流驱动,径向悬浮力控制采用三相逆变器提供电流驱动,由一块径向充磁的环形永磁体同时提供轴向、径向偏磁磁通,同时引入一组二片式六极径向轴向双磁极面结构,大幅增大了径向磁极面积,提高磁轴承的径向承载力,并且在保证径向承载力的情况下,减小轴向尺寸。轴承集合了交流驱动、永磁偏置及径向-轴向联合控制等优点。理论分析和有限元仿真证明,磁轴承的结构设计更加合理,对磁悬浮传动系统向大功率、微型化方向发展具有一定意义。
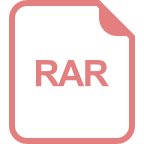
PyGuide-working.rar
使用python编写的基于genesis2000的cam-guide软件。genesis2000接口用的python3.0 可以自己找网上的2.0改一改,很简单
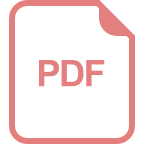
主要的边缘智能参考架构-arm汇编语言官方手册
(3)新型基础设施平台
5G 新型基础设施平台的基础是网络功能虚拟化(NFV)和软件定义网络(SDN)
技术。IMT2020(5G)推进组发布的《5G网络技术架构白皮书》认为,通过软件
与硬件的分离,NFV 为 5G网络提供更具弹性的基础设施平台,组件化的网络功
能模块实现控制面功能可重构,并对通用硬件资源实现按需分配和动态伸缩,以
达到优化资源利用率。SDN技术实现控制功能和转发功能的分离,这有利于网络
控制平面从全局视角来感知和调度网络资源。NFV和 SDN技术的进步成熟,也给
移动边缘计算打下坚实基础。
2.3 主要的边缘智能参考架构
边缘智能的一些产业联盟及标准化组织作为产业服务机构,会持续推出边缘
计算技术参考架构,本节总结主要标准化组织的参考架构。
欧洲电信标准化协会(ETSI) 2016年 4 月 18日发布了与 MEC相关的重量级
标准,对 MEC的七大业务场景作了规范和详细描述,主要包括智能移动视频加速、
监控视频流分析、AR、密集计算辅助、在企业专网之中的应用、车联网、物联网
网关业务等七大场景。
此外,还发布了发布三份与 MEC相关的技术规范,分别涉及 MEC 术语、技术
需求及用例、MEC框架与参考架构。
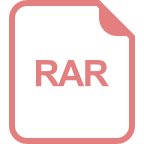
[C#]文件中转站程序及源码
在网上看到一款名为“DropPoint文件复制中转站”的工具,于是自己尝试仿写一下。并且添加一个移动文件的功能。
用来提高复制粘贴文件效率的工具,它会给你一个临时中转悬浮框,只需要将一处或多处想要复制的文件拖拽到这个悬浮框,再一次性拖拽至目的地文件夹,就能高效完成复制粘贴及移动文件。
支持拖拽多个文件到悬浮框,并显示文件数量
将悬浮窗内的文件往目标文件夹拖拽即可实现复制,适用于整理文件
主要的功能实现:
1、实现文件拖拽功能,将文件或者文件夹拖拽到软件上
2、实现文件拖拽出来,将文件或目录拖拽到指定的位置
3、实现多文件添加,包含目录及文件
4、添加软件透明背景、软件置顶、文件计数
最新推荐
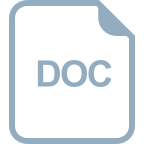
电子英汉词典设计 课程设计报告
通过设计一个简单的电子英汉词典系统,学生们将学习如何运用结构化程序设计方法解决实际问题,同时提升数据结构和算法的应用能力,以及文档编写和程序调试技巧。 **设计内容与要求** 1. **课程设计目的**: - ...
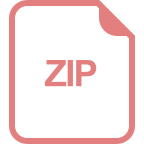
毕业设计基于单片机的室内有害气体检测系统源码+论文(高分毕设)
毕业设计基于单片机的室内有害气体检测系统源码+论文(高分毕设)毕业设计基于单片机的室内有害气体检测系统源码毕业设计基于单片机的室内有害气体检测系统源码+论文,含有代码注释,简单部署使用。结合毕业设计文档进行理解。
有害气体检测报警系统分为四个子系统:主控制系统,室内气体检测系统,信息交互可视化系统与信息处理识别反馈系统。有害气体检测报警系统如图2-1所示,主控系统为核心,通过控制室内检测系统采集数据之后进行数据回传。回传的数据经过信息处理识别反馈系统及预处理后进行可视化展现与指标判断,并且最终根据所得数据判断是否需要预警,完成规避风险的功能。
有害气体检测未来研究趋势:
室内有害气体检测在现代社会中变得愈发重要,关乎人们的健康和居住环境的质量。随着城市化的加速和室内空间的日益密集,有害气体如CO、CO2、甲醛等的排放成为一项不可忽视的问题。以下通过了解国内外在这一领域的最新研究,为基于单片机的室内有害气体检测报警系统的设计提供依据。
(1)数据处理与算法:
国内的研究人员致力于改进数据处理算法,以更有效地处理大量的监测数据。智能算法的引入,如机器学习和人工智能,有助于提高对室内空气质
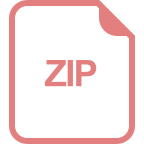
易语言例程:用易核心支持库打造功能丰富的IE浏览框
资源摘要信息:"易语言-易核心支持库实现功能完善的IE浏览框"
易语言是一种简单易学的编程语言,主要面向中文用户。它提供了大量的库和组件,使得开发者能够快速开发各种应用程序。在易语言中,通过调用易核心支持库,可以实现功能完善的IE浏览框。IE浏览框,顾名思义,就是能够在一个应用程序窗口内嵌入一个Internet Explorer浏览器控件,从而实现网页浏览的功能。
易核心支持库是易语言中的一个重要组件,它提供了对IE浏览器核心的调用接口,使得开发者能够在易语言环境下使用IE浏览器的功能。通过这种方式,开发者可以创建一个具有完整功能的IE浏览器实例,它不仅能够显示网页,还能够支持各种浏览器操作,如前进、后退、刷新、停止等,并且还能够响应各种事件,如页面加载完成、链接点击等。
在易语言中实现IE浏览框,通常需要以下几个步骤:
1. 引入易核心支持库:首先需要在易语言的开发环境中引入易核心支持库,这样才能在程序中使用库提供的功能。
2. 创建浏览器控件:使用易核心支持库提供的API,创建一个浏览器控件实例。在这个过程中,可以设置控件的初始大小、位置等属性。
3. 加载网页:将浏览器控件与一个网页地址关联起来,即可在控件中加载显示网页内容。
4. 控制浏览器行为:通过易核心支持库提供的接口,可以控制浏览器的行为,如前进、后退、刷新页面等。同时,也可以响应浏览器事件,实现自定义的交互逻辑。
5. 调试和优化:在开发完成后,需要对IE浏览框进行调试,确保其在不同的操作和网页内容下均能够正常工作。对于性能和兼容性的问题需要进行相应的优化处理。
易语言的易核心支持库使得在易语言环境下实现IE浏览框变得非常方便,它极大地降低了开发难度,并且提高了开发效率。由于易语言的易用性,即使是初学者也能够在短时间内学会如何创建和操作IE浏览框,实现网页浏览的功能。
需要注意的是,由于IE浏览器已经逐渐被微软边缘浏览器(Microsoft Edge)所替代,使用IE核心的技术未来可能面临兼容性和安全性的挑战。因此,在实际开发中,开发者应考虑到这一点,并根据需求选择合适的浏览器控件实现技术。
此外,易语言虽然简化了编程过程,但其在功能上可能不如主流的编程语言(如C++, Java等)强大,且社区和技术支持相比其他语言可能较为有限,这些都是在选择易语言作为开发工具时需要考虑的因素。
文件名列表中的“IE类”可能是指包含实现IE浏览框功能的类库或者示例代码。在易语言中,类库是一组封装好的代码模块,其中包含了各种功能的实现。通过在易语言项目中引用这些类库,开发者可以简化开发过程,快速实现特定功能。而示例代码则为开发者提供了具体的实现参考,帮助理解和学习如何使用易核心支持库来创建IE浏览框。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
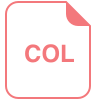
STM32F407ZG引脚功能深度剖析:掌握引脚分布与配置的秘密(全面解读)
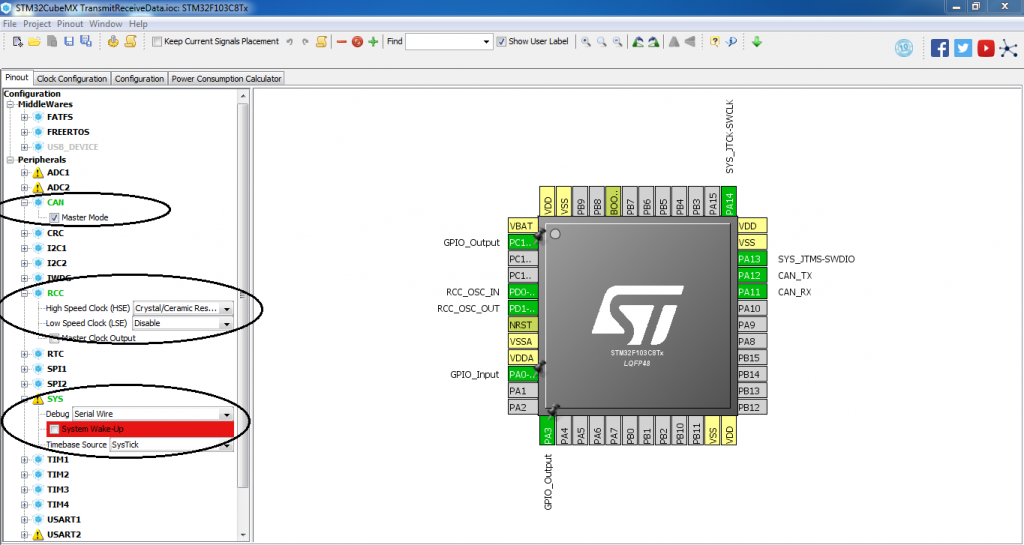
# 摘要
本文全面介绍了STM32F407ZG微控制器的引脚特性、功能、配置和应用。首先概述了该芯片的引脚布局,然后详细探讨了标准外设、高级控制以及特殊功能引脚的不同配置和使用方法。在此基础上,文章深入分析了引脚模式配置、高级配置技巧,并提供了实际应用案例,如LED控制和串口通信。在设计方面,阐述了引脚布局策略、多层板设计及高密度引脚应用的解决方案。最后,介绍

给出文档中问题的答案代码
您提到的是需要编写MATLAB代码来实现文档中的实验任务。以下是根据文档内容编写的MATLAB代码示例:
```matlab
% 上机2 实验代码
% 读取输入图像
inputImage = imread('your_face_image.jpg'); % 替换为您的图像文件路径
if size(inputImage, 1) < 1024 || size(inputImage, 2) < 1024
error('图像尺寸必须大于1024x1024');
end
% 将彩色图像转换为灰度图像
grayImage = rgb2gray(inputImage);
% 调整图像大小为5
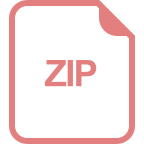
Docker构建与运行Next.js应用的指南
资源摘要信息:"rivoltafilippo-next-main"
在探讨“rivoltafilippo-next-main”这一资源时,首先要从标题“rivoltafilippo-next”入手。这个标题可能是某一项目、代码库或应用的命名,结合描述中提到的Docker构建和运行命令,我们可以推断这是一个基于Docker的Node.js应用,特别是使用了Next.js框架的项目。Next.js是一个流行的React框架,用于服务器端渲染和静态网站生成。
描述部分提供了构建和运行基于Docker的Next.js应用的具体命令:
1. `docker build`命令用于创建一个新的Docker镜像。在构建镜像的过程中,开发者可以定义Dockerfile文件,该文件是一个文本文件,包含了创建Docker镜像所需的指令集。通过使用`-t`参数,用户可以为生成的镜像指定一个标签,这里的标签是`my-next-js-app`,意味着构建的镜像将被标记为`my-next-js-app`,方便后续的识别和引用。
2. `docker run`命令则用于运行一个Docker容器,即基于镜像启动一个实例。在这个命令中,`-p 3000:3000`参数指示Docker将容器内的3000端口映射到宿主机的3000端口,这样做通常是为了让宿主机能够访问容器内运行的应用。`my-next-js-app`是容器运行时使用的镜像名称,这个名称应该与构建时指定的标签一致。
最后,我们注意到资源包含了“TypeScript”这一标签,这表明项目可能使用了TypeScript语言。TypeScript是JavaScript的一个超集,它添加了静态类型定义的特性,能够帮助开发者更容易地维护和扩展代码,尤其是在大型项目中。
结合资源名称“rivoltafilippo-next-main”,我们可以推测这是项目的主目录或主仓库。通常情况下,开发者会将项目的源代码、配置文件、构建脚本等放在一个主要的目录中,这个目录通常命名为“main”或“src”等,以便于管理和维护。
综上所述,我们可以总结出以下几个重要的知识点:
- Docker容器和镜像的概念以及它们之间的关系:Docker镜像是静态的只读模板,而Docker容器是从镜像实例化的动态运行环境。
- `docker build`命令的使用方法和作用:这个命令用于创建新的Docker镜像,通常需要一个Dockerfile来指定构建的指令和环境。
- `docker run`命令的使用方法和作用:该命令用于根据镜像启动一个或多个容器实例,并可指定端口映射等运行参数。
- Next.js框架的特点:Next.js是一个支持服务器端渲染和静态网站生成的React框架,适合构建现代的Web应用。
- TypeScript的作用和优势:TypeScript是JavaScript的一个超集,它提供了静态类型检查等特性,有助于提高代码质量和可维护性。
- 项目资源命名习惯:通常项目会有一个主目录,用来存放项目的源代码和核心配置文件,以便于项目的版本控制和团队协作。
以上内容基于给定的信息进行了深入的分析,为理解该项目的构建、运行方式以及技术栈提供了基础。在实际开发中,开发者应当参考更详细的文档和指南,以更高效地管理和部署基于Docker和TypeScript的Next.js项目。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
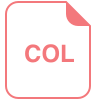
【热传递模型的终极指南】:掌握分类、仿真设计、优化与故障诊断的18大秘诀

# 摘要
热传递模型在工程和物理学中占有重要地位,对于提高热交换效率和散热设计至关重要。本文系统性地介绍了热传递模型的基础知识、分类以及在实际中的应用案例。文章详细阐述了导热、对流换热以及辐射传热的基本原理,并对不同类型的热传递模型进行了分类,包括稳态与非稳态模型、一维到三维模型和线性与非线性模型。通过仿真设计章节,文章展示了如何选择合适的仿真软件、构建几何模型、设置材料属性和

python经典题型和解题代码
Python的经典题型通常涵盖了基础语法、数据结构、算法、函数式编程、文件操作、异常处理以及网络爬虫等内容。以下是一些常见的题目及其简单示例:
1. **基础题**:
- 示例:打印九九乘法表
```python
for i in range(1, 10):
print(f"{i} * {i} = {i*i}")
```
2. **数据结构**:
- 示例:实现队列(使用列表)
```python
class Queue:
def __init__(self):