c语言实现金山打字游戏
时间: 2023-07-13 12:07:49 浏览: 325
金山打字游戏是一款经典的打字练习软件,通过反复练习可以提高打字速度和准确性。以下是一个简单的C语言实现金山打字游戏的示例代码,供您参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define WORDS_NUM 10 // 单词数量
#define WORDS_LEN 20 // 单词长度
char words[WORDS_NUM][WORDS_LEN] = { // 单词列表
"apple", "banana", "cherry", "orange", "lemon",
"grape", "watermelon", "peach", "pear", "pineapple"
};
void shuffle_words() { // 打乱单词顺序
srand(time(NULL));
for (int i = WORDS_NUM - 1; i > 0; i--) {
int j = rand() % (i + 1);
char temp[WORDS_LEN];
strcpy(temp, words[i]);
strcpy(words[i], words[j]);
strcpy(words[j], temp);
}
}
int main() {
char input[WORDS_LEN];
int score = 0;
shuffle_words(); // 打乱单词顺序
for (int i = 0; i < WORDS_NUM; i++) {
printf("Type the word: %s\n", words[i]);
scanf("%s", input);
if (strcmp(input, words[i]) == 0) {
printf("Correct!\n");
score++;
} else {
printf("Wrong! The correct word is: %s\n", words[i]);
}
}
printf("Your score is: %d\n", score);
return 0;
}
```
该程序首先定义了一个包含10个单词的数组,并通过`shuffle_words()`函数打乱了单词顺序。接着,在主函数中循环输出每个单词并等待用户输入,如果用户输入的单词与正确答案相同则加分,否则输出正确答案并继续下一轮。最后输出用户的得分。
该程序仅为示例代码,您可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐











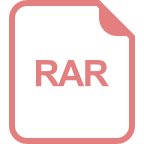
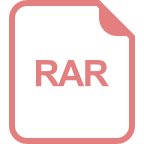
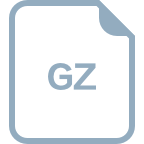



